Intro
In this program, we’ll create a Python program to find the minimum and maximum elements in a Binary Search Tree (BST). A Binary Search Tree is a binary tree where each node has a value, and for any node, all the values in the left subtree are less than the node’s value, and all the values in the right subtree are greater than the node’s value.
Problem statement
Given a Binary Search Tree, we need to find the minimum and maximum elements present in the tree.
Python Program to Find Minimum and Maximum Element in Binary Search Tree
class TreeNode: def __init__(self, value): self.value = value self.left = None self.right = None def find_min(node): if node is None: return None while node.left is not None: node = node.left return node.value def find_max(node): if node is None: return None while node.right is not None: node = node.right return node.value root = TreeNode(8) root.left = TreeNode(3) root.right = TreeNode(10) root.left.left = TreeNode(1) root.left.right = TreeNode(6) root.right.right = TreeNode(14) root.left.right.left = TreeNode(4) root.left.right.right = TreeNode(7) print("Minimum element:", find_min(root)) print("Maximum element:", find_max(root))
How it works
- We start by defining a
TreeNode
class to create nodes of the Binary Search Tree. Each node has avalue
,left
child, andright
child. - The
find_min
function takes a node as an argument and traverses to the leftmost node of the tree to find the minimum value. - The
find_max
function takes a node as an argument and traverses to the rightmost node of the tree to find the maximum value. - In the example tree, we create a BST and then use the
find_min
andfind_max
functions to find and print the minimum and maximum elements.
Input / Output
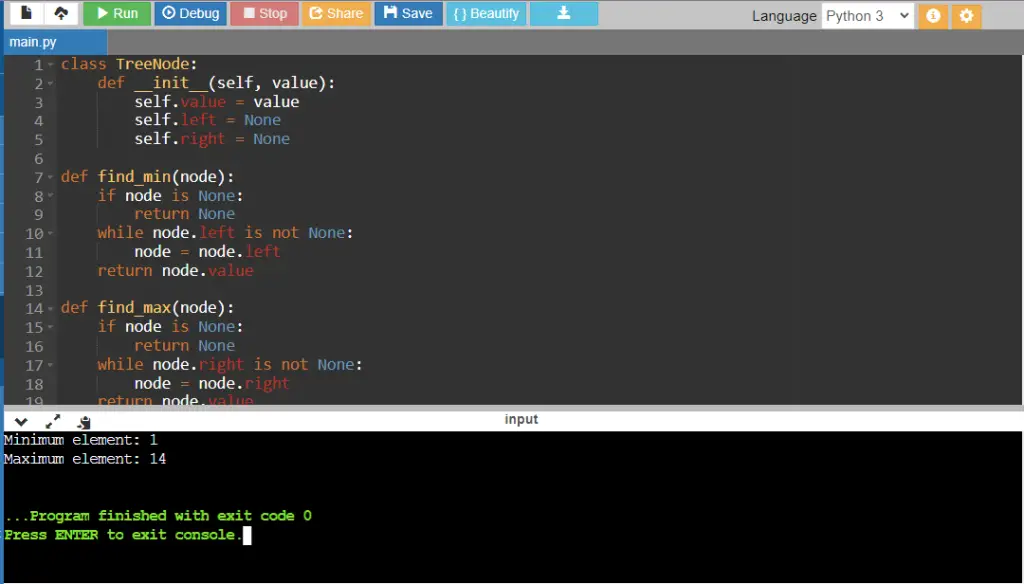