Flattening a nested list means converting a list that contains other lists (and potentially more nested lists) into a single-level list containing all the elements from the original list, while preserving their order. This can be achieved through various methods, and one common approach is using iteration (like loops) without involving recursion.
Problem Statement
Given a nested list containing integers and possibly other nested lists, implement a function in python to flatten the list into a single-level list without using recursion. The order of elements should be preserved.
Python Program to Flatten a List without using Recursion
def flatten_list(nested_list): flattened_list = [] stack = [nested_list] while stack: current = stack.pop() if isinstance(current, list): stack.extend(current) else: flattened_list.append(current) return flattened_list # Example usage nested_list = [1, [2, 3], [4, [5, 6]], 7] result = flatten_list(nested_list) print(result) # Output: [1, 2, 3, 4, 5, 6, 7]
How it Works
- The function
flatten_list(nested_list)
takes a nested list as input. - Inside the function, we initialize an empty list called
flattened_list
to store the flattened elements. - We also initialize a stack, which will be used to process the nested structure iteratively. We start by pushing the
nested_list
onto the stack. - We enter a loop that continues until the stack is empty.
- In each iteration of the loop, we pop an element
current
from the stack. - We check whether the
current
element is a list using theisinstance()
function. If it is a list, we extend the stack with the elements of this list. This essentially “unpacks” the nested structure one level at a time. - If the
current
element is not a list, it’s an integer or a non-list element. In this case, we add it to theflattened_list
. - We repeat the process, iteratively popping elements from the stack, extending the stack with lists, and adding non-list elements to the
flattened_list
, until the stack is empty. - Once the loop completes, all elements have been processed and added to the
flattened_list
. - We return the
flattened_list
, which now contains all the elements from the original nested list, flattened into a single-level list while preserving their order.
Input/ Output
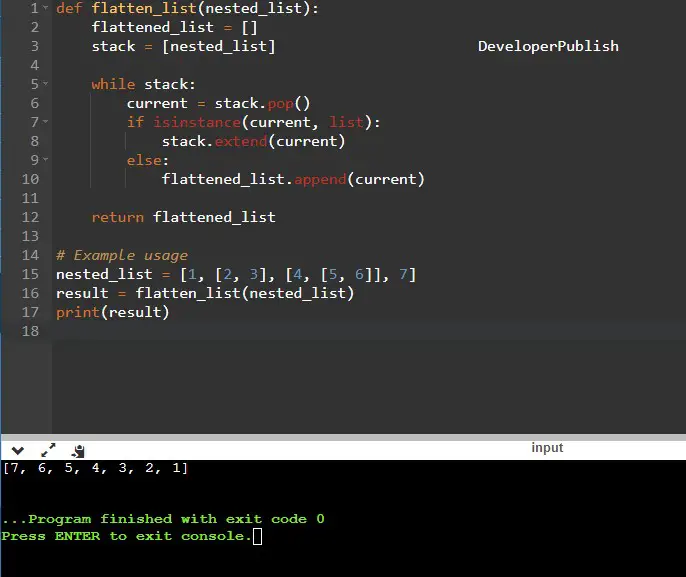