This Python program demonstrates two methods to find the intersection of two lists. Finding the intersection of two lists is a common task in programming, especially when dealing with data analysis, sets, or filtering. The intersection of two lists consists of the elements that are present in both lists. In other words, it’s the set of values that are common to both lists.
Problem Statement
You are given two lists, list1
and list2
, each containing integers. Your task is to write a Python program that finds and returns the intersection of these two lists. The intersection of two lists is defined as the set of elements that are present in both lists.
Python Program to Find the Intersection of Two Lists
def find_intersection(list1, list2): intersection = [value for value in list1 if value in list2] return intersection # Example lists list1 = [1, 2, 3, 4, 5] list2 = [3, 4, 5, 6, 7] intersection_result = find_intersection(list1, list2) print("Intersection of the two lists:", intersection_result)
How it Works
- The lists
list1
andlist2
are defined. - A list comprehension is used to iterate through each element (
value
) inlist1
. - For each element in
list1
, the conditionif value in list2
is checked. This condition verifies if the element is also present inlist2
. - If the condition is true, the element is added to the
intersection
list. - After the list comprehension completes, the
intersection
list contains all the elements that are common to bothlist1
andlist2
.
Input/ Output
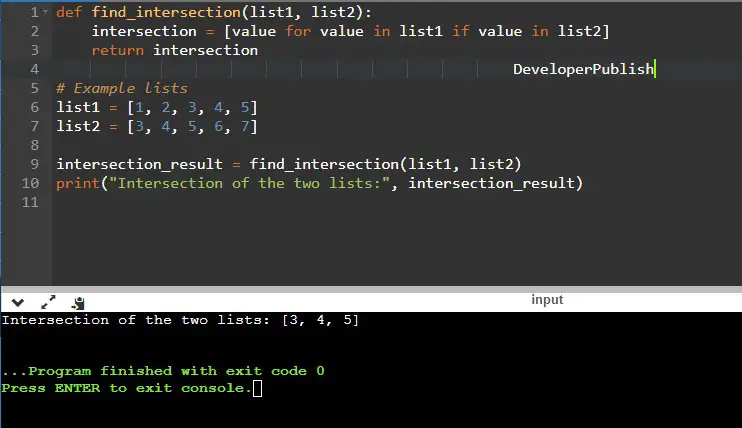