Linked lists are fundamental data structures in computer science, offering a dynamic way to organize and manage data. In this context, we will explore a Python program that constructs and exhibits a linked list, demonstrating the principles of this data structure.
Problem Statement
Compose a Python program to create and exhibit a simple linked list. The program should illustrate the process of building a linked list by sequentially adding elements, and then display the linked list’s contents.
Python Program to Create and Display Linked List
class Node: def __init__(self, data): self.data = data self.next = None class LinkedList: def __init__(self): self.head = None def append(self, data): new_node = Node(data) if self.head is None: self.head = new_node return current = self.head while current.next: current = current.next current.next = new_node def display(self): current = self.head while current: print(current.data, end=" -> ") current = current.next print("None") # Create a linked list my_linked_list = LinkedList() my_linked_list.append(3) my_linked_list.append(7) my_linked_list.append(10) # Display the linked list print("Linked List:") my_linked_list.display()
Input / Output
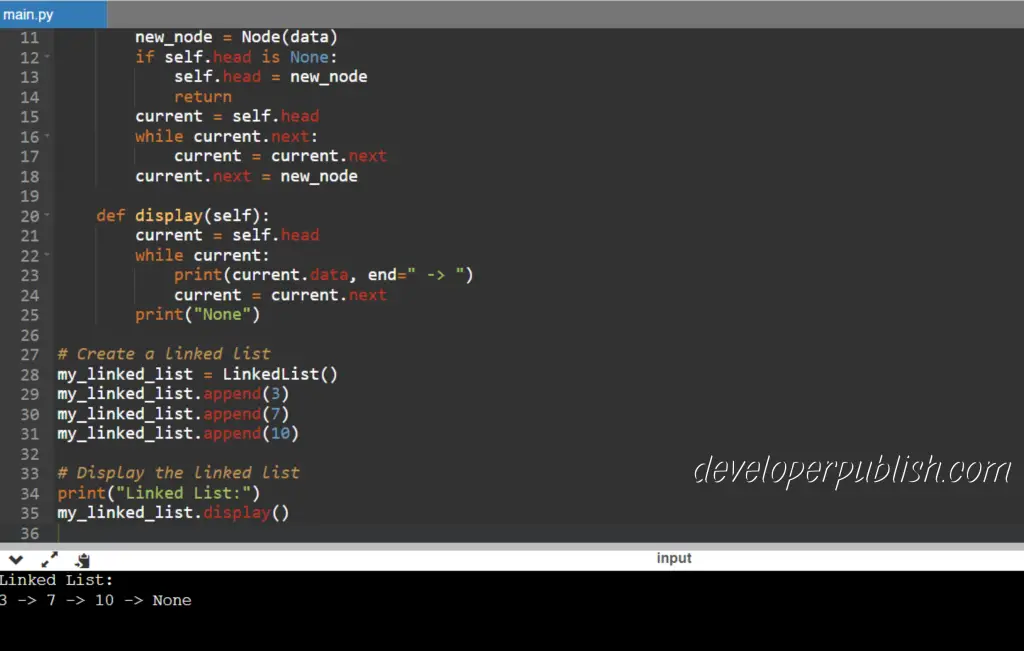