String comparison is a fundamental operation in programming. This program showcases how to create a Python program to find the larger of two input strings without relying on built-in functions.
Problem Statement
Write a Python program that takes two strings as input and determines which one is larger in terms of character length, without using any built-in length-related functions.
Python Program to Find the Larger String without Using Built-in Functions
def find_larger_string(str1, str2): len1 = 0 len2 = 0 for char in str1: len1 += 1 for char in str2: len2 += 1 if len1 > len2: return str1 elif len2 > len1: return str2 else: return "Both strings have the same length." # Input string1 = input("Enter the first string: ") string2 = input("Enter the second string: ") # Finding the larger string larger_string = find_larger_string(string1, string2) # Output print("The larger string is:", larger_string)
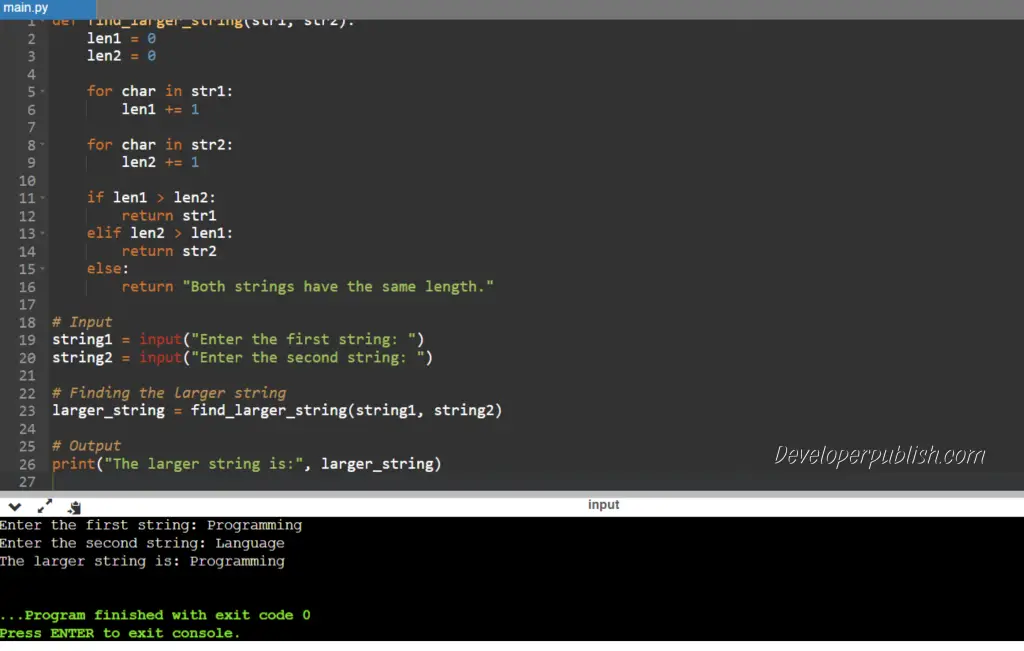