Text files often contain a mix of characters, numbers, and symbols. This program demonstrates how to create a Python program that reads a text file, extracts and identifies numbers within the text, and presents them to the user.
Problem Statement
Write a Python program that takes the name of a text file as input, reads the contents of the file, and extracts and displays all the numbers found in the text.
Python Program to Extract Numbers from Text File
import re def extract_numbers_from_file(filename): try: with open(filename, 'r') as file: contents = file.read() numbers = re.findall(r'\d+', contents) return numbers except FileNotFoundError: return [] # Input file_name = "sample.txt" # Provide the name of your text file here # Extracting numbers from file number_list = extract_numbers_from_file(file_name) # Output if number_list: print("Numbers extracted from the file:", ', '.join(number_list)) else: print("No numbers found in the file.")
Input / Output
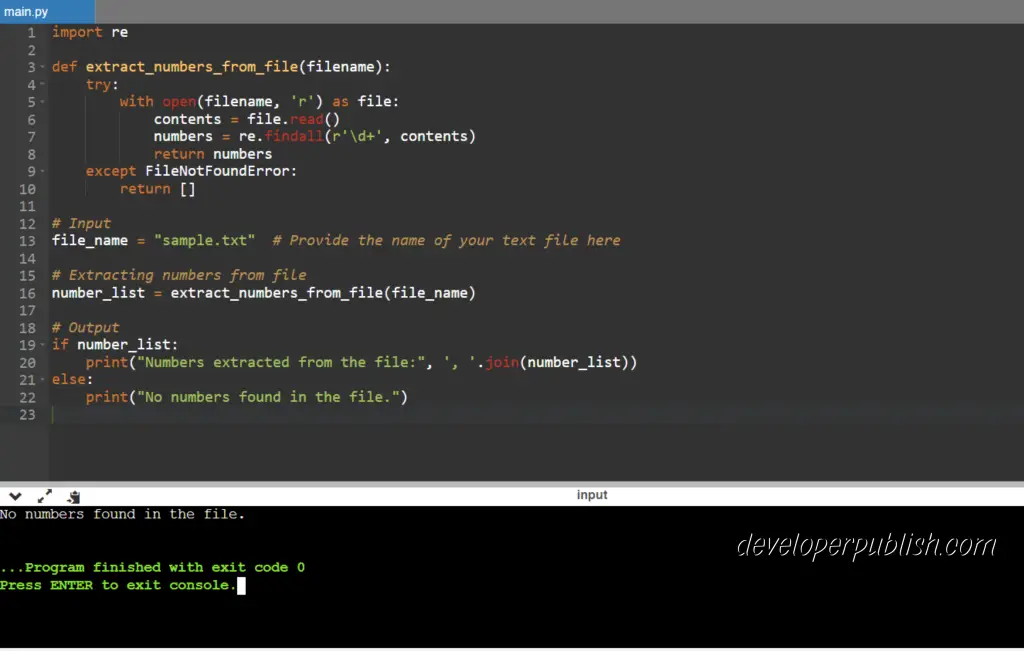