Text files often contain various characters, including blank spaces. This python program demonstrates how to create a Python program that reads a text file and counts the number of blank spaces present in its contents.
Problem Statement
Write a Python program that takes the name of a text file as input, reads its contents, and counts the occurrences of blank spaces within the text.
Python Program to Count the Number of Blank Spaces in a Text File
def count_blank_spaces(filename): try: with open(filename, 'r') as file: contents = file.read() blank_space_count = contents.count(' ') return blank_space_count except FileNotFoundError: return 0 # Input file_name = input("Enter the name of the text file: ") # Counting blank spaces in the file blank_space_count = count_blank_spaces(file_name) # Output print("Number of blank spaces in the file:", blank_space_count)
Input / Output
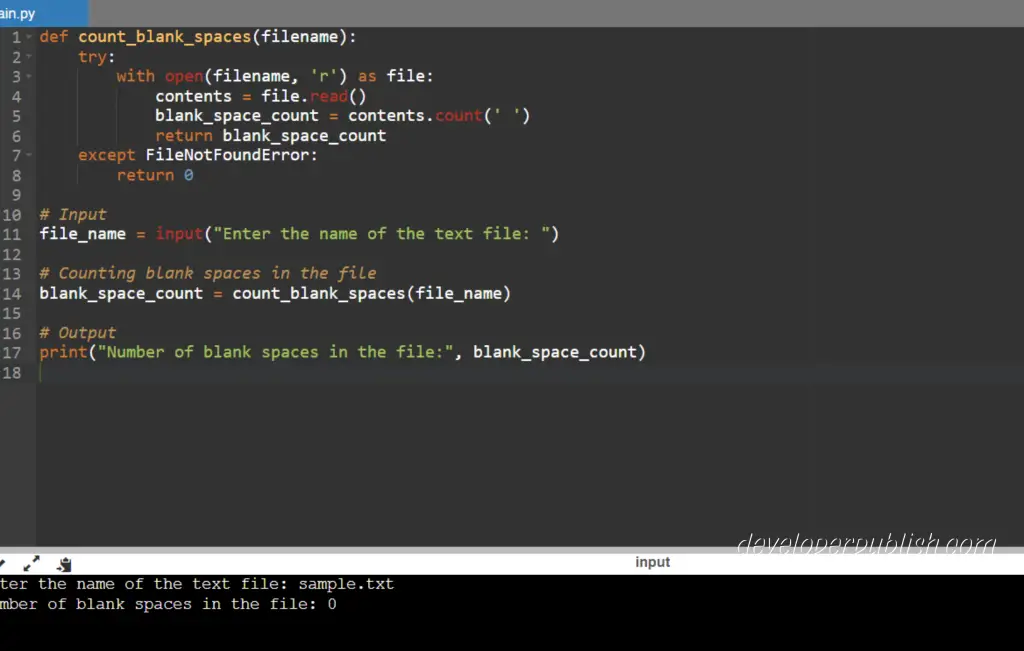