Text files often contain various characters, including blank spaces. This python program demonstrates how to create a Python program that reads a text file and counts the number of blank spaces present in its contents.
Problem Statement
Write a Python program that takes the names of two text files as input, reads the content of one file, and appends that content to the end of another file.
Python Program to Append the Content of One File to the End of Another File
def append_file_contents(source_file, target_file): try: with open(source_file, 'r') as source: source_contents = source.read() with open(target_file, 'a') as target: target.write(source_contents) return True except FileNotFoundError: return False # Input source_file_name = input("Enter the name of the source file: ") target_file_name = input("Enter the name of the target file: ") # Appending content to the target file success = append_file_contents(source_file_name, target_file_name) # Output if success: print("Content appended successfully.") else: print("Source file not found. Content could not be appended.")
Input / Output
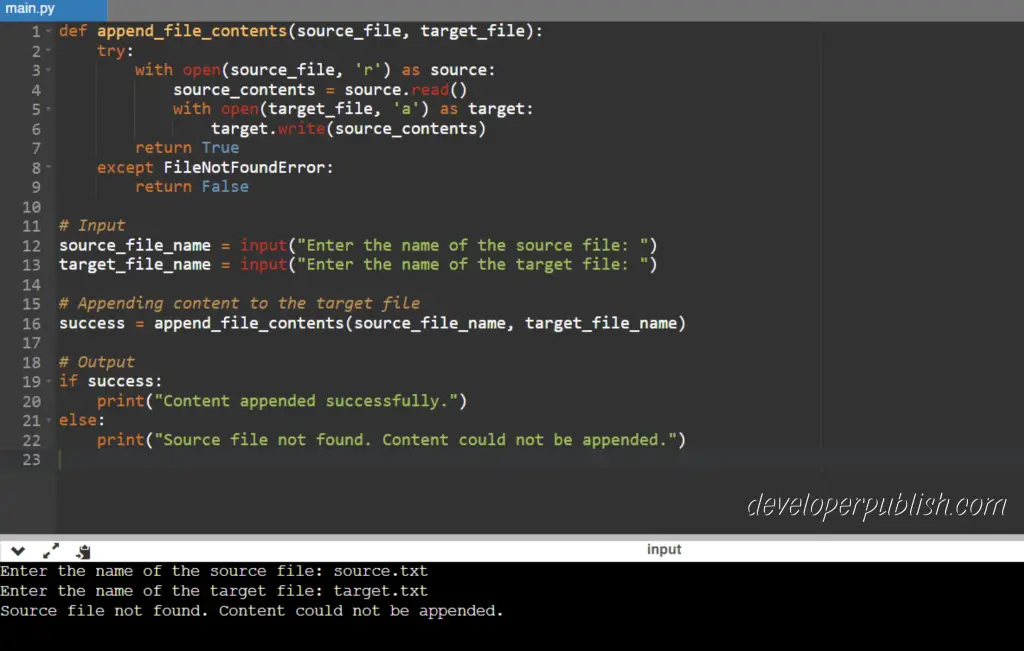