This Python program will help you find all the prime numbers within a given range. A prime number is a positive integer greater than 1 that has no positive divisors other than 1 and itself.
Problem Statement
Given a range of numbers, we need to find all the prime numbers within that range.
Python Program to Find Prime Numbers in a Given Range
import math def is_prime(num): if num < 2: return False for i in range(2, int(math.sqrt(num)) + 1): if num % i == 0: return False return True def find_prime_numbers(start, end): prime_numbers = [] for num in range(start, end + 1): if is_prime(num): prime_numbers.append(num) return prime_numbers # Get input from the user start = int(input("Enter the starting number of the range: ")) end = int(input("Enter the ending number of the range: ")) # Find prime numbers within the given range prime_numbers = find_prime_numbers(start, end) # Print the prime numbers print("Prime numbers within the given range are:") print(prime_numbers)
How it works
The program will take two inputs: start
and end
, representing the starting and ending numbers of the range, respectively. It will iterate through each number in the range and check if it is a prime number.
To determine if a number num
is prime, the program will check if num
is divisible by any number from 2 to the square root of num
. If num
is divisible by any number within this range, it is not prime. Otherwise, it is prime.
The program will store all the prime numbers in a list and finally print the list.
Input / Output
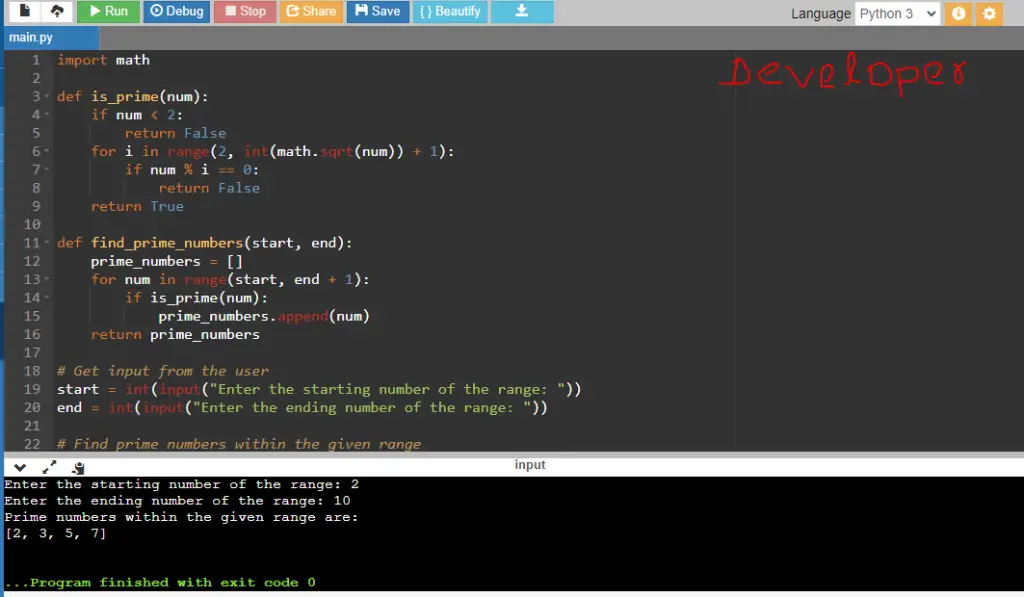