This python program will validate whether a given date is valid or not, based on the input provided by the user.
Problem Statement
Given a date in the format of day, month, and year, we need to check whether the date is valid or not. If the date is valid, the program will display a message indicating that the date is valid. Otherwise, it will display a message stating that the date is invalid.
Python Program to Validate a Date
def is_valid_date(day, month, year): # List of maximum days in each month max_days = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31] # Check if it's a leap year if year % 4 == 0 and (year % 100 != 0 or year % 400 == 0): max_days[1] = 29 # Check if the month is valid if month < 1 or month > 12: return False # Check if the day is valid if day < 1 or day > max_days[month - 1]: return False return True # Main program date_input = input("Enter a date (day, month, year): ") day, month, year = map(int, date_input.split(',')) if is_valid_date(day, month, year): print("The date is valid.") else: print("The date is invalid.")
How it works
The program defines a function called is_valid_date()
to check the validity of the given date. It takes three parameters: day
, month
, and year
. The function first creates a list called max_days
representing the maximum number of days in each month. By default, February has 28 days.
Next, the function checks if the given year is a leap year. A leap year occurs if the year is divisible by 4 and not divisible by 100, or if it is divisible by 400. If the year is a leap year, it updates the maximum number of days in February to 29.
After that, the function checks if the month is within the valid range of 1 to 12. If the month is invalid, it returns False
.
Finally, the function checks if the given day is within the valid range for the given month. It retrieves the maximum number of days for the corresponding month from the max_days
list and compares it with the given day. If the day is invalid, it returns False
. Otherwise, it returns True
to indicate that the date is valid.
In the main program, the user is prompted to enter a date in the format “day, month, year”. The input is then split and converted into integers using the map()
function. The program calls the is_valid_date()
function with the provided day, month, and year. If the function returns True
, it prints “The date is valid.” Otherwise, it prints “The date is invalid.”
Input / Output
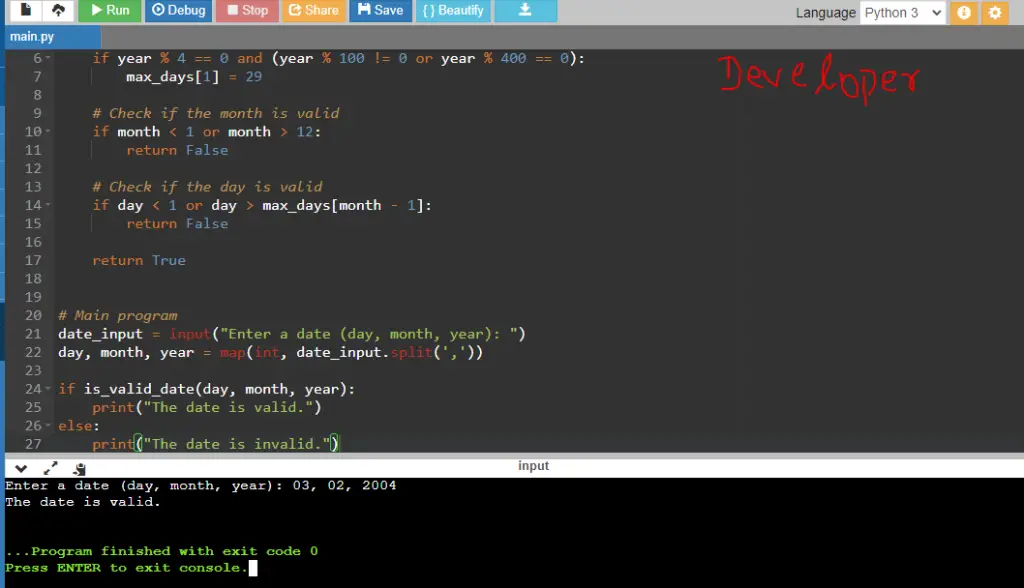