Odd palindrome numbers are numbers that remain the same when read from left to right and from right to left. This program demonstrates how to create a Python program to find all odd palindrome numbers within a specified range without utilizing recursion.
Problem Statement
Write a Python program that takes a range as input and identifies all the odd palindrome numbers within that range, without using recursion.
Python Program to Find All Odd Palindrome Numbers in a Range without using Recursion
def is_palindrome(number): num_str = str(number) return num_str == num_str[::-1] def find_odd_palindromes_in_range(start, end): odd_palindromes = [] for num in range(start, end + 1): if num % 2 == 1 and is_palindrome(num): odd_palindromes.append(num) return odd_palindromes # Input start_range = int(input("Enter the start of the range: ")) end_range = int(input("Enter the end of the range: ")) # Finding odd palindrome numbers odd_palindromes = find_odd_palindromes_in_range(start_range, end_range) # Output if odd_palindromes: print("Odd palindrome numbers in the range:", odd_palindromes) else: print("No odd palindrome numbers found in the given range.")
Input / Output
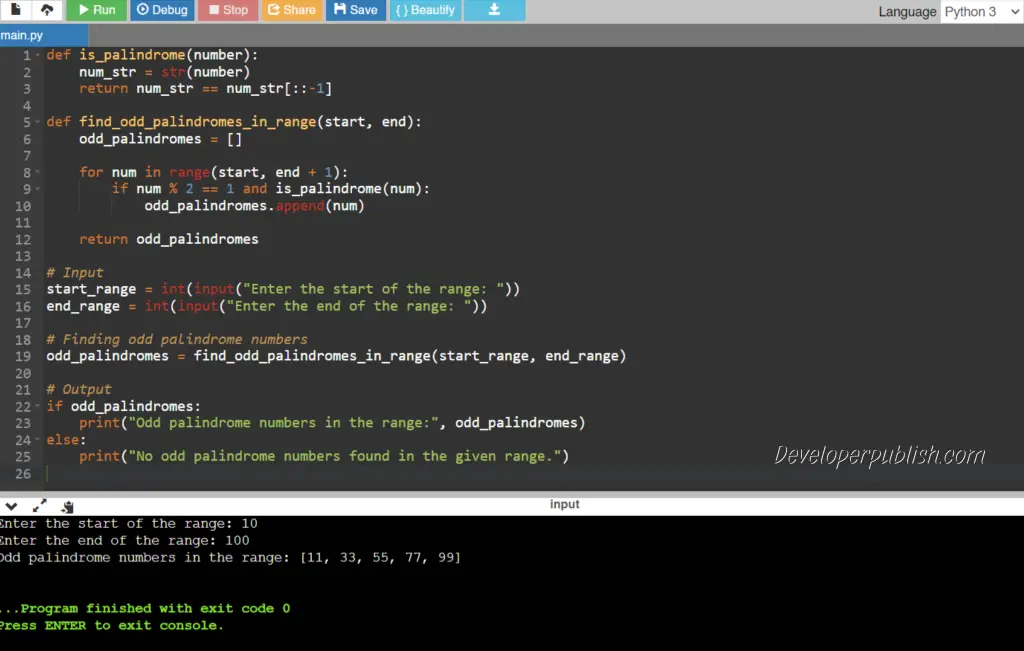