This Python program uses sets to count the number of vowels in a given string.
Problem Statement:
You are tasked with writing a Python program that takes a string as input and counts the number of vowels (both uppercase and lowercase) present in the string using sets.
Python Program to Count Number of Vowels in a String using Sets
def count_vowels(input_string): vowels = {'a', 'e', 'i', 'o', 'u', 'A', 'E', 'I', 'O', 'U'} vowel_count = 0 for char in input_string: if char in vowels: vowel_count += 1 return vowel_count # Input from the user user_input = input("Enter a string: ") result = count_vowels(user_input) print("Number of vowels:", result)
How it Works:
- The program defines a function
count_vowels
that takes an input string as its parameter. - A set named
vowels
is created containing all lowercase and uppercase vowels. - The program iterates through each character in the input string using a
for
loop. - If the current character is found in the
vowels
set, thevowel_count
is incremented by 1. - After iterating through all characters, the
vowel_count
is returned. - The user is prompted to enter a string.
- The
count_vowels
function is called with the user-input string, and the result is printed as the number of vowels in the string.
Input/Output:
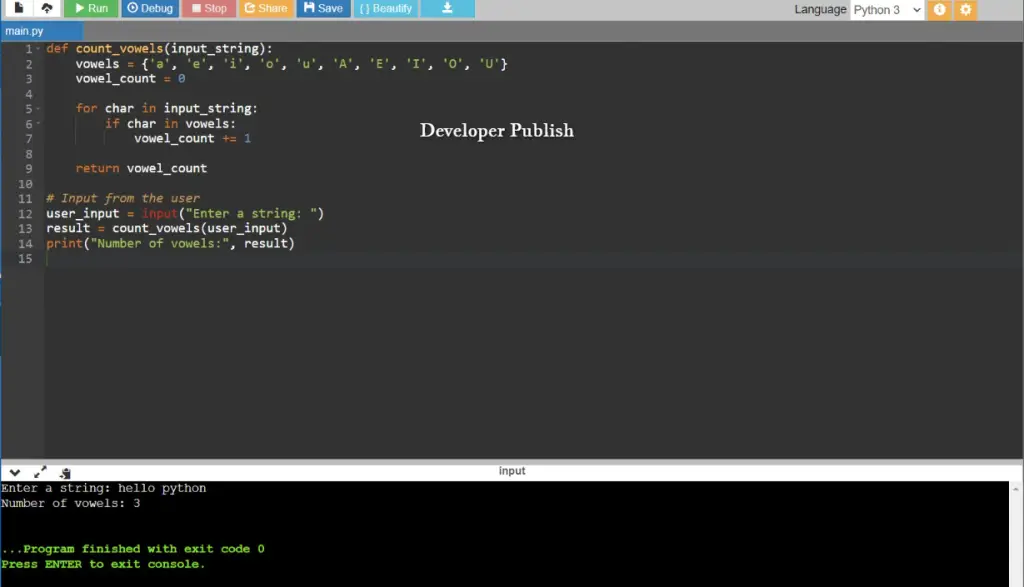