In this program, we will create a Python script to concatenate two dictionaries. Dictionaries are data structures that store key-value pairs. Concatenating dictionaries means combining the key-value pairs from two dictionaries into a single dictionary.
Problem Statement:
Given two dictionaries, you need to write a python program to concatenate them into a single dictionary.
Python Program to Concatenate Two Dictionaries
def concatenate_dictionaries(dict1, dict2): concatenated_dict = dict1.copy() # Create a copy of the first dictionary concatenated_dict.update(dict2) # Update the copy with the contents of the second dictionary return concatenated_dict # Input dictionaries dict1 = {'a': 1, 'b': 2} dict2 = {'c': 3, 'd': 4} # Concatenate dictionaries result_dict = concatenate_dictionaries(dict1, dict2) print("Concatenated Dictionary:", result_dict)
How it Works:
- We define a function
concatenate_dictionaries
that takes two dictionaries (dict1
anddict2
) as arguments. - Inside the function, we create a copy of
dict1
using the.copy()
method. This ensures that we preserve the original contents ofdict1
without modifying it. - We then use the
.update()
method to update the copy (concatenated_dict
) with the key-value pairs fromdict2
. This merges the contents of the two dictionaries. - The function returns the concatenated dictionary.
- We provide two example dictionaries (
dict1
anddict2
) and call theconcatenate_dictionaries
function to concatenate them. - Finally, we print the concatenated dictionary.
Input/Output:
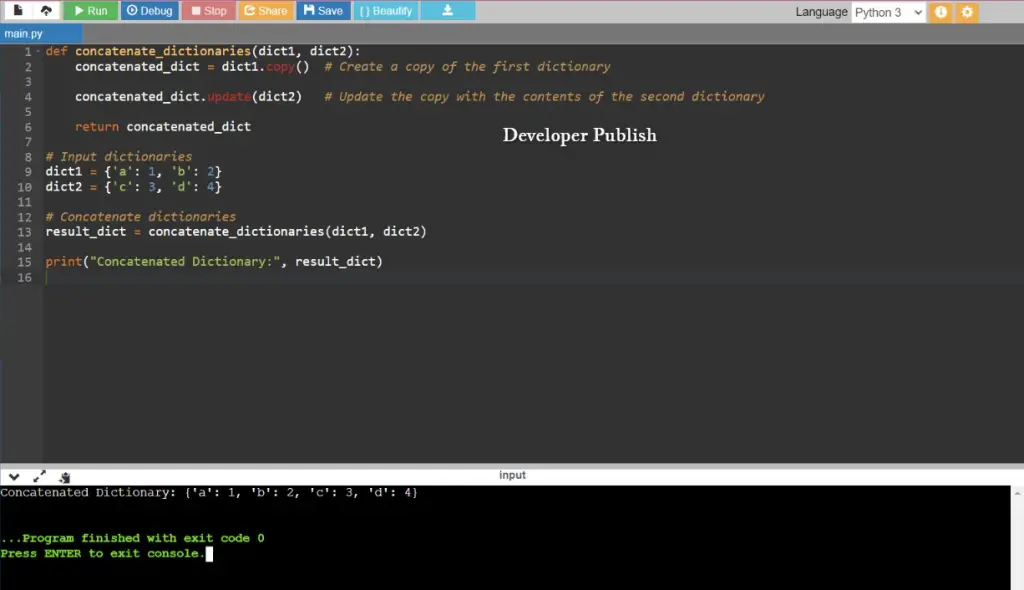