Capitalizing the first letter of each word in a file is a common text manipulation task in programming. It involves processing the content of a text file, identifying the words within it, and modifying them to have their initial letters capitalized. This process can be useful for various applications, such as cleaning up text, formatting titles, or enhancing the readability of content.
Problem Statement
You are given a text file containing sentences. Your task is to write a python program that reads the content of the file, capitalizes the first letter of each word in every sentence, and then writes the modified content back to the same file.
Python Program to Capitalize First Letter of Each Word in a File
def capitalize_words_in_file(file_path): try: with open(file_path, 'r') as file: content = file.read() capitalized_content = ' '.join(word.capitalize() for word in content.split()) with open(file_path, 'w') as file: file.write(capitalized_content) print("First letter of each word capitalized and saved to the file.") except FileNotFoundError: print(f"File '{file_path}' not found.") except Exception as e: print("An error occurred:", e) # Replace 'input.txt' with the path to your input file file_path = 'input.txt' capitalize_words_in_file(file_path)
How it Works
- Function Definition:The program starts by defining a function called
capitalize_words_in_file
that takes a single argumentfile_path
, which is the path to the input file. - Reading the File Content:Inside a
try
block, the program attempts to open the specified file (file_path
) in read mode using a context manager (with
statement). The content of the file is read and stored in the variablecontent
. - Capitalizing Words:The content is split into a list of words using the
split()
method. Then, a generator expression is used to iterate through each word in the list and capitalize the first letter of each word using thecapitalize()
method. The generator expression creates a new capitalized word list. Finally, thejoin()
method is used to join the capitalized words back into a single string with spaces. - Writing Modified Content:The program opens the same file (
file_path
) again, but this time in write mode. This will overwrite the original content of the file. The capitalized content is then written back to the file. - Handling Errors:The program includes error handling using
try
andexcept
blocks. If the specified file is not found, aFileNotFoundError
will be caught and an error message will be displayed. Any other exceptions will be caught by the genericException
case, and an error message with the specific exception information will be displayed. - Calling the Function:At the end of the program, the function
capitalize_words_in_file
is called with thefile_path
argument set to'input.txt'
. You should replace'input.txt'
with the actual path to your input file.
Input/ Output
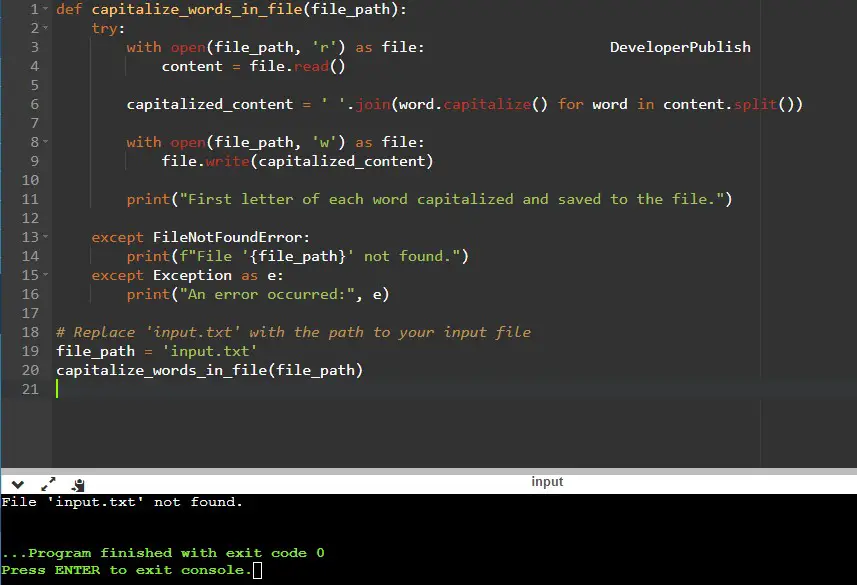