This C# program is designed to calculate and display the factors of a specified positive integer. Factors are the numbers that evenly divide the given number without leaving a remainder.
Problem Statement
You are tasked with writing a program that finds all the factors of a given positive integer number. Factors are the positive integers that evenly divide the given number without leaving a remainder.
C# Program to Find the Factors of the Given Number
using System; class Program { static void Main() { Console.Write("Enter a number: "); int number = int.Parse(Console.ReadLine()); Console.WriteLine("Factors of " + number + " are:"); FindFactors(number); Console.ReadLine(); } static void FindFactors(int num) { for (int i = 1; i <= num; i++) { if (num % i == 0) { Console.WriteLine(i); } } } }
How it Works
- User Input: The first step is to get the value of
n
from the user or from some data source. - Initialization: Initialize a loop variable, usually called
i
, to start at 1. This variable will be used to check each number from 1 ton
to see if it’s a factor. - Factor Checking Loop: Enter a loop that iterates from
i
= 1 toi
=n
. For each iteration of the loop, do the following:a. Check ifn
is divisible byi
(i.e.,n % i == 0
). If the remainder of the division is 0, it means thati
is a factor ofn
.b. Ifi
is a factor, print or store the value ofi
because it’s one of the factors ofn
.c. Increment the value ofi
to move to the next number in the loop. - Loop Termination: Continue the loop until
i
reachesn
. This ensures that all numbers from 1 ton
are checked for being factors. - Display Factors: After the loop completes, you have found all the factors of
n
. You can display these factors to the user or use them for further calculations. - Program Ends: The program ends, and you have successfully found and processed the factors of the given number
n
.
Input/ Output
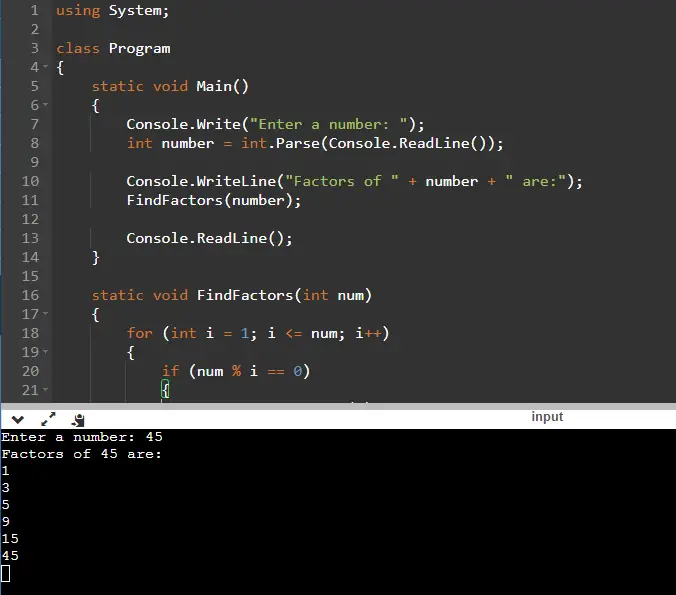