This C program checks whether a given number is a palindrome or not, where the number is entered by the user. The program then prints a message to the screen indicating whether the number is a palindrome or not.
Problem Statement
Write a C program that checks whether a given number is a palindrome or not, where the number is entered by the user. The program should prompt the user to enter the number, determine whether it is a palindrome or not, and print a message to the screen indicating whether the number is a palindrome or not.
Solution
#include <stdio.h> int main() { int n, reversed_number = 0, remainder, original_number; // Get the value of n from user printf("Enter a positive integer: "); scanf("%d", &n); original_number = n; // Reverse the number while (n != 0) { remainder = n % 10; reversed_number = reversed_number * 10 + remainder; n /= 10; } // Check if the number is a palindrome or not if (original_number == reversed_number) printf("%d is a palindrome number.", original_number); else printf("%d is not a palindrome number.", original_number); return 0; }
Output
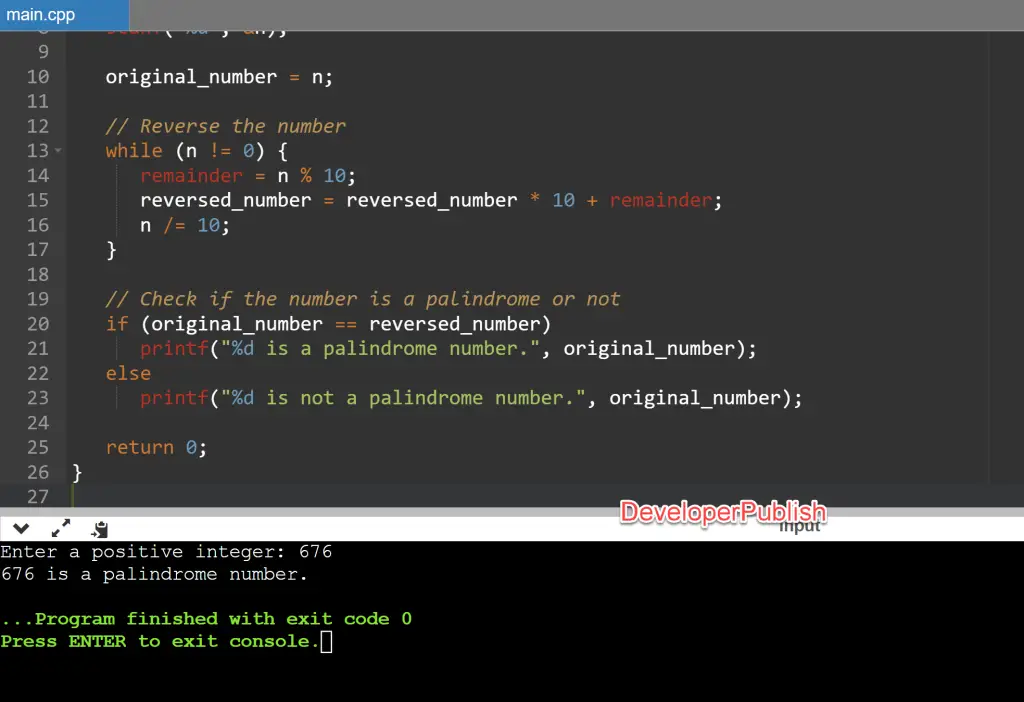
Explanation
The program starts by declaring variables to hold the value of the number entered by the user (n), the reversed number (reversed_number), the remainder of the number when divided by 10 (remainder), and the original number (original_number).
The program then prompts the user to enter a positive integer, and stores the value in the n variable. The program also stores the original number in the original_number variable for comparison later.
Next, the program reverses the number using a while loop, similar to the previous example. In each iteration of the loop, the program calculates the remainder of the number when divided by 10 using the modulo operator (%), and adds it to the reversed number variable multiplied by 10 (to shift the existing digits one place to the left). The program then divides the number by 10 to remove the last digit.
After the loop, the program checks whether the original number is equal to the reversed number. If they are equal, the program prints a message saying that the number is a palindrome. Otherwise, the program prints a message saying that the number is not a palindrome.
Finally, the program exits.
Conclusion
This C program demonstrates how to check whether a given number is a palindrome or not. It prompts the user to enter the number, reverses the number, and compares the original number with the reversed number to determine whether the number is a palindrome or not. The program then prints a message to the screen indicating whether the number is a palindrome or not.