In this program, we will write a C program to read a file and capitalize the first letter of each word present in the file. The program will read the content of the file, modify it by capitalizing the first letter of each word, and then write the modified content back to the file.
Problem statement
Write a C program that reads the contents of a file, capitalizes the first letter of each word in the file, and writes the modified content back to the same file.
C Program to Capitalize First Letter of Each Word in a File
#include <stdio.h> #include <ctype.h> void capitalizeFirstLetter(char *str) { int capitalize = 1; while (*str) { if (isspace(*str)) { capitalize = 1; } else if (capitalize) { *str = toupper(*str); capitalize = 0; } str++; } } int main() { FILE *file; char filename[100]; char buffer[1000]; printf("Enter the name of the file: "); scanf("%s", filename); // Open the file in read mode file = fopen(filename, "r"); if (file == NULL) { printf("Unable to open the file.\n"); return 1; } // Read the content of the file fgets(buffer, sizeof(buffer), file); // Close the file fclose(file); // Capitalize the first letter of each word capitalizeFirstLetter(buffer); // Open the file in write mode file = fopen(filename, "w"); if (file == NULL) { printf("Unable to open the file.\n"); return 1; } // Write the modified content back to the file fputs(buffer, file); // Close the file fclose(file); printf("Successfully capitalized the first letter of each word in the file.\n"); return 0; }
How it works
- The program starts by including the necessary header files:
stdio.h
for standard input/output functions andctype.h
for character handling functions. - The
capitalizeFirstLetter
function is defined to capitalize the first letter of each word in a given string. It takes a character array (string) as input and modifies it in-place. - In the
main
function, a file pointerfile
and character arraysfilename
andbuffer
are declared. - The user is prompted to enter the name of the file to be processed.
- The file is opened in read mode using
fopen
function. If the file does not exist or cannot be opened, an error message is displayed, and the program terminates. - The content of the file is read using
fgets
function and stored in thebuffer
array. - The file is closed using
fclose
function. - The
capitalizeFirstLetter
function is called with thebuffer
array as an argument to capitalize the first letter of each word. - The file is opened again, but this time in write mode.
- If the file cannot be opened in write mode, an error message is displayed, and the program terminates.
- The modified content stored in the
buffer
array is written back to the file usingfputs
function. - The file is closed.
- Finally, a success message is displayed indicating that the first letter of each word has been capitalized in the file.
Input / output
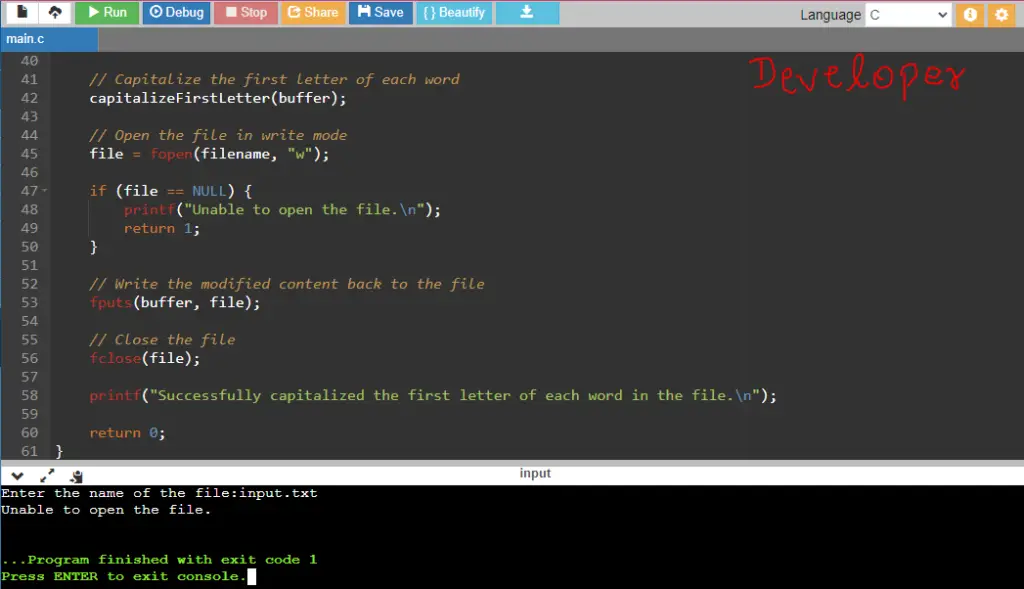