This Python program implements a deque (double-ended queue) using a list. A deque allows elements to be added or removed from both ends efficiently. The Deque
class defines methods for common deque operations:
A double-ended queue, or deque, is a data structure that allows insertion and removal of elements from both ends with constant time complexity. It can be thought of as a hybrid of a stack and a queue, providing versatile functionality for various use cases.
Problem Statement
Implement a deque data structure in Python, allowing operations such as insertion and removal of elements from both ends efficiently.
Python Program to Implement Dequeue
class Deque: def __init__(self): self.items = [] def is_empty(self): return len(self.items) == 0 def add_front(self, item): self.items.insert(0, item) def add_rear(self, item): self.items.append(item) def remove_front(self): if not self.is_empty(): return self.items.pop(0) return None def remove_rear(self): if not self.is_empty(): return self.items.pop() return None def size(self): return len(self.items) # Example usage deque = Deque() deque.add_front(10) deque.add_rear(20) deque.add_front(5) print("Deque size:", deque.size()) print("Removed front:", deque.remove_front()) print("Removed rear:", deque.remove_rear()) print("Is deque empty?", deque.is_empty())
How it Works
The program defines a class Deque
that encapsulates the deque data structure. It uses a Python list (self.items
) to store elements. The methods add_front
, add_rear
, remove_front
, remove_rear
, size
, and is_empty
provide the core functionality of the deque.
add_front(item)
: Inserts an item at the front of the deque.add_rear(item)
: Inserts an item at the rear of the deque.remove_front()
: Removes and returns an item from the front of the deque.remove_rear()
: Removes and returns an item from the rear of the deque.size()
: Returns the number of items in the deque.is_empty()
: Returns whether the deque is empty or not.
Input/Output
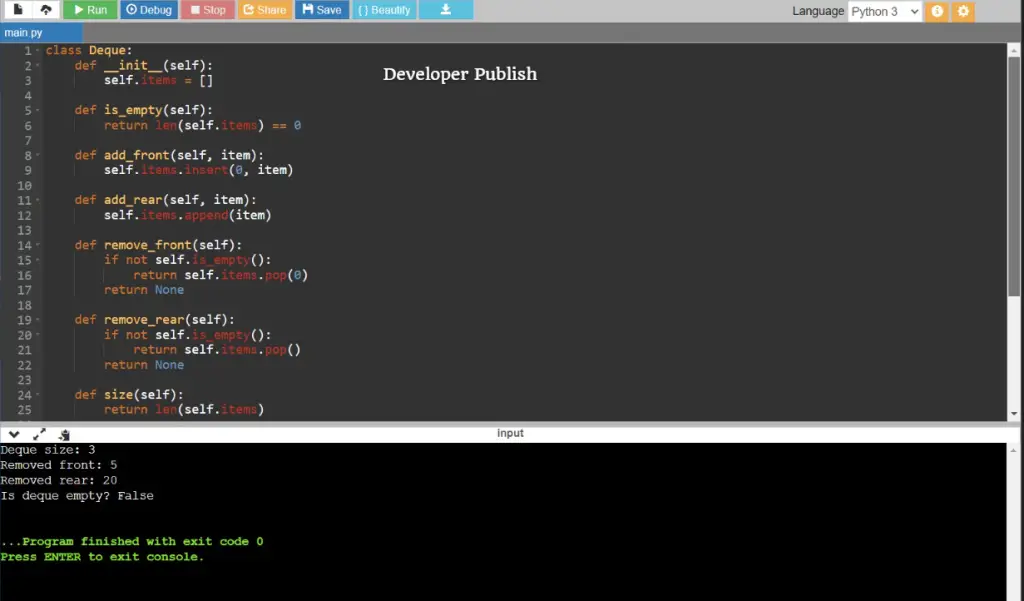