Dictionaries in Python are key-value pairs that provide efficient data storage and retrieval. This program demonstrates how to create a Python program to check whether a given key exists in a dictionary.
Problem Statement
Write a Python program that takes a key and a dictionary as input and determines whether the given key exists in the dictionary or not.
Python Program to Check if a Key Exists in a Dictionary
def key_exists(dictionary, key): return key in dictionary # Input (Creating a dictionary) sample_dict = { 'name': 'John', 'age': 30, 'city': 'New York' } key_to_check = input("Enter the key to check: ") # Checking if the key exists if key_exists(sample_dict, key_to_check): print(f"The key '{key_to_check}' exists in the dictionary.") else: print(f"The key '{key_to_check}' does not exist in the dictionary.")
Input / Output
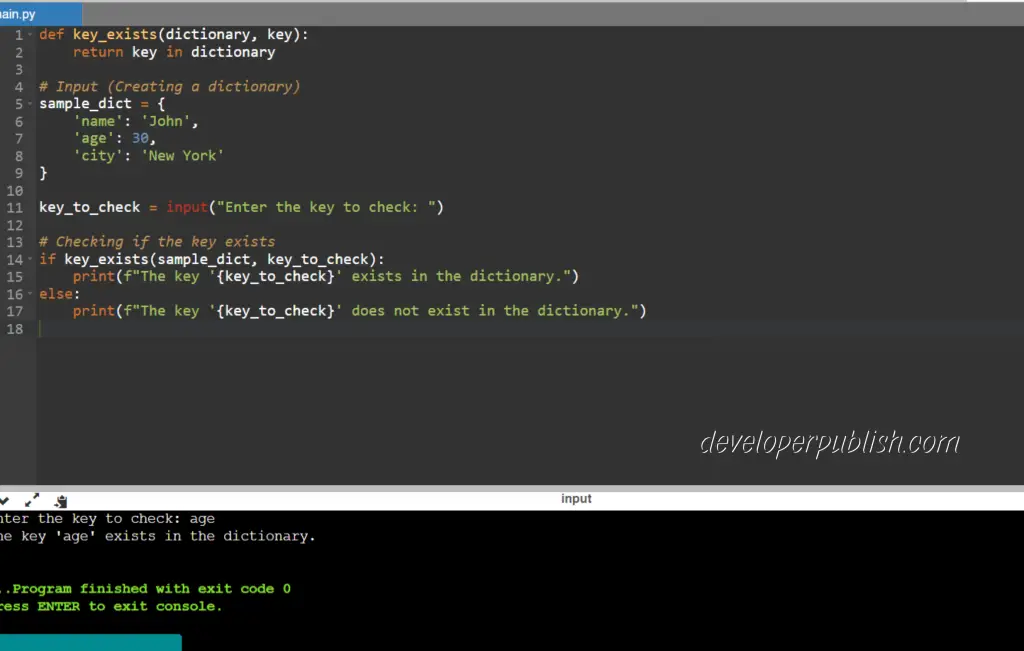