In Python, a dictionary is a powerful data structure that allows you to store and manage data in key-value pairs. Each key in a dictionary maps to a specific value, and you can use keys to access corresponding values quickly. Dictionaries are versatile and can store various types of data, including numbers.
Problem Statement
You are tasked with creating a program that manages a dictionary containing names of numbers and their corresponding numerical values.
Python Program to Create Dictionary that Contains Number
# Creating a dictionary with numbers number_dict = { "one": 1, "two": 2, "three": 3, "four": 4, "five": 5 } # Accessing values from the dictionary print("Number corresponding to 'two':", number_dict["two"]) print("Number corresponding to 'four':", number_dict["four"]) # Adding more entries to the dictionary number_dict["six"] = 6 number_dict["seven"] = 7 # Printing the updated dictionary print("Updated Dictionary:", number_dict)
How it Works
- Creating the Dictionary: Here, we create a dictionary named
number_dict
with initial key-value pairs. The keys are strings (“one”, “two”, etc.) representing the names of the numbers, and the values are corresponding numerical values. - Accessing Values: These lines demonstrate how to access and print values from the dictionary using the keys. It prints the values associated with the keys “two” and “four”.
- Adding Entries: Here, we add two more key-value pairs to the dictionary. We use the syntax
dictionary[key] = value
to add or update entries in the dictionary. - Printing the Updated Dictionary: Finally, we print the entire updated dictionary, including the new entries added in the previous step.
Input/ Output
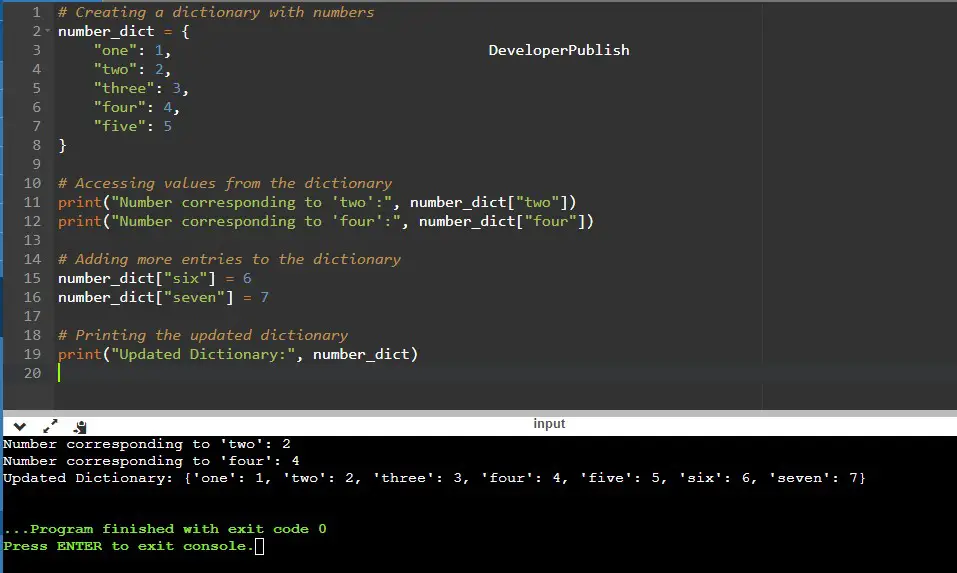