In this Python program, we’ll create a class that represents a list. The class will have methods to append elements, delete elements, and display the elements of the list. This program demonstrates the use of classes and methods to manipulate a list-like data structure.
Problem Statement:
Create a Python program that defines a class CustomList
. This class should have methods to append elements to the list, delete elements from the list, and display the elements of the list.
Python Program to Append, Delete and Display Elements of a List using Classes
class CustomList: def __init__(self): self.data = [] def append_element(self, element): self.data.append(element) def delete_element(self, element): if element in self.data: self.data.remove(element) else: print(f"{element} not found in the list.") def display_elements(self): print("List Elements:", self.data) # Create an instance of the CustomList class my_list = CustomList() # Append elements to the list my_list.append_element(10) my_list.append_element(20) my_list.append_element(30) # Display the initial list elements my_list.display_elements() # Delete an element from the list my_list.delete_element(20) # Display the updated list elements my_list.display_elements()
How it Works:
- We define a class named
CustomList
with three methods:append_element
,delete_element
, anddisplay_elements
. - The
__init__
method initializes an empty listdata
when an instance of the class is created. - The
append_element
method takes an element as an argument and appends it to thedata
list. - The
delete_element
method takes an element as an argument, checks if it exists in thedata
list, and removes it if found. - The
display_elements
method simply prints the elements of thedata
list. - We create an instance of the
CustomList
class calledmy_list
. - We append three elements to the list using the
append_element
method. - We display the elements of the list using the
display_elements
method. - We delete an element using the
delete_element
method. - We again display the updated elements of the list.
Input/Output:
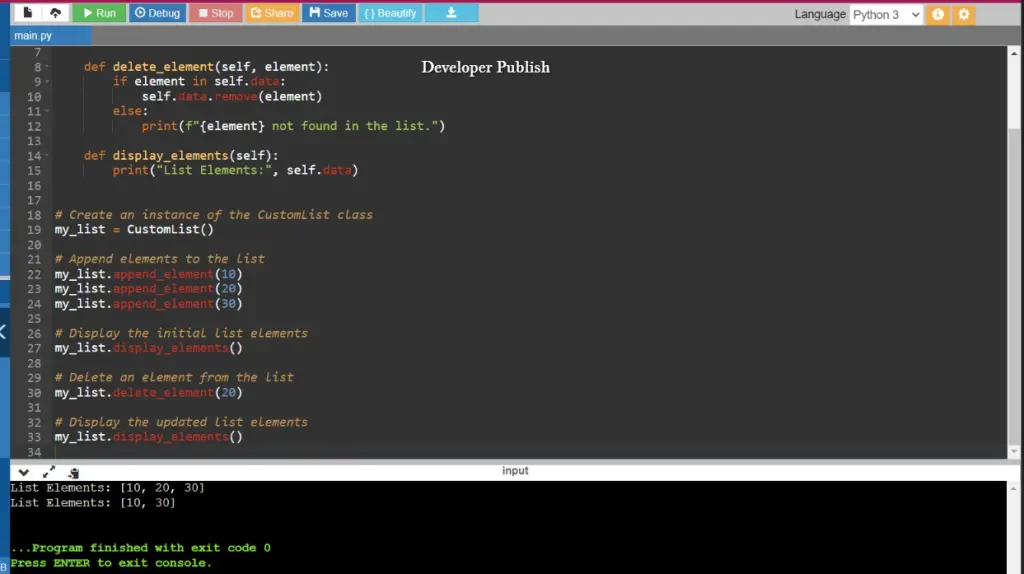