In this program, we will write a C program to find the sum of each row and column of a matrix.
Problem Statement
We need to take a matrix as input from the user and calculate the sum of each row and column of the matrix. Then, we have to display the sum of each row and column on the screen.
Solution:
#include <stdio.h> int main() { int rows, columns, i, j, sum = 0; // Get the number of rows and columns from the user printf("Enter the number of rows: "); scanf("%d", &rows); printf("Enter the number of columns: "); scanf("%d", &columns); int matrix[rows][columns]; // Get the elements of the matrix from the user printf("Enter the elements of the matrix: \n"); for (i = 0; i < rows; i++) { for (j = 0; j < columns; j++) { scanf("%d", &matrix[i][j]); } } // Calculate the sum of each row printf("Sum of each row: "); for (i = 0; i < rows; i++) { sum = 0; for (j = 0; j < columns; j++) { sum += matrix[i][j]; } printf("%d ", sum); } // Calculate the sum of each column printf("\nSum of each column: "); for (i = 0; i < columns; i++) { sum = 0; for (j = 0; j < rows; j++) { sum += matrix[j][i]; } printf("%d ", sum); } return 0; }
Output
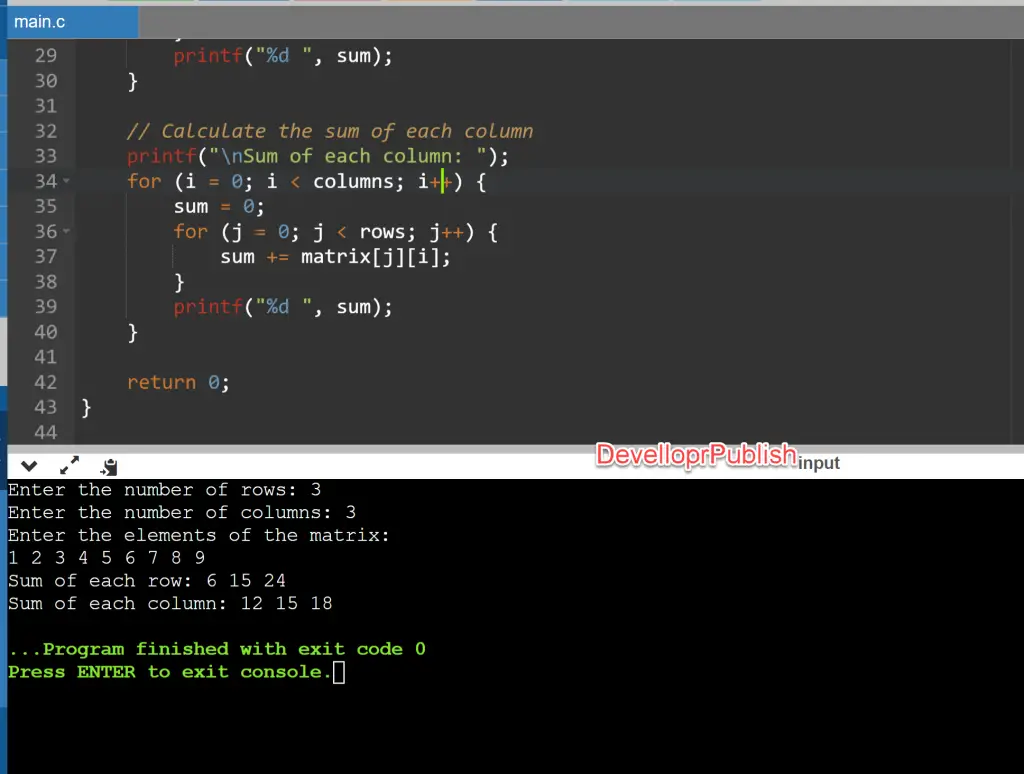
Explanation:
- We first include the standard input-output library
stdio.h
in the program using the#include
preprocessor directive. - Then, we declare the main function using the
int main()
syntax. - We declare four variables
rows
,columns
,i
, andj
as integers, and initialize thesum
variable to 0. - We use the
printf
function to prompt the user to enter the number of rows and columns of the matrix. - We use the
scanf
function to read the number of rows and columns entered by the user and store them in therows
andcolumns
variables respectively. - We declare a two-dimensional integer array
matrix
withrows
rows andcolumns
columns. - We use a nested for loop to read the elements of the matrix entered by the user and store them in the
matrix
array. - We use another nested for loop to calculate the sum of each row of the matrix. In each iteration of the outer loop, we reset the
sum
variable to 0. In the inner loop, we add the elements of the current row to thesum
variable. Finally, we use theprintf
function to display the sum of the current row on the screen. - We use another nested for loop to calculate the sum of each column of the matrix. In each iteration of the outer loop, we reset the
sum
variable to 0. In the inner loop, we add the elements of the current column to thesum
variable. Finally, we use theprintf
function to display the sum of the current column on the screen.
Conclusion:
We have successfully written a C program to find the sum of each row and column of a matrix. This program can be useful in various applications where we need to perform matrix operations.