This C program calculates the length of a given string.
Problem Statement
Write a program that calculates the average of three numbers entered by the user.
The program should perform the following steps:
- Prompt the user to enter three numbers.
- Read the three numbers from the user.
- Calculate the average of the three numbers.
- Display the calculated average on the screen.
Example:
Enter the first number: 5 Enter the second number: 7 Enter the third number: 3 The average of the three numbers is: 5.0
Requirements:
- The program should be written in C programming language.
- The user input and output should be handled through the console.
- The average should be displayed with one decimal place.
- The program should handle both integer and floating-point input.
You can use the provided problem statement as a guide to write the code. Remember to include necessary input validation and error handling to ensure the program functions correctly.
C Program to Find the Length of the String
#include <stdio.h> int main() { double num1, num2, num3; double average; printf("Enter the first number: "); scanf("%lf", &num1); printf("Enter the second number: "); scanf("%lf", &num2); printf("Enter the third number: "); scanf("%lf", &num3); average = (num1 + num2 + num3) / 3; printf("The average of the three numbers is: %.1lf\n", average); return 0; }
How it Works
- The program starts by prompting the user to enter three numbers. This is done using
printf
statements to display messages to the user on the console. - The program then uses
scanf
to read the three numbers entered by the user. The numbers are stored in variables for further processing. - Next, the program calculates the average of the three numbers. It does this by adding the three numbers together and dividing the sum by 3. The average is stored in a variable.
- Finally, the program displays the calculated average on the console using a
printf
statement. The average is printed with one decimal place by using the format specifier%.1f
.
Here’s an example of how the program would execute:
Enter the first number: 5 Enter the second number: 7 Enter the third number: 3 The average of the three numbers is: 5.0
In this example, the user enters the numbers 5, 7, and 3. The program calculates the average as (5 + 7 + 3) / 3 = 5.0 and displays it on the console.
Please note that the program assumes valid input from the user. It doesn’t handle scenarios where the user enters invalid input, such as non-numeric values. Adding input validation and error handling is important to ensure the program behaves as expected in all situations.
Input / Output
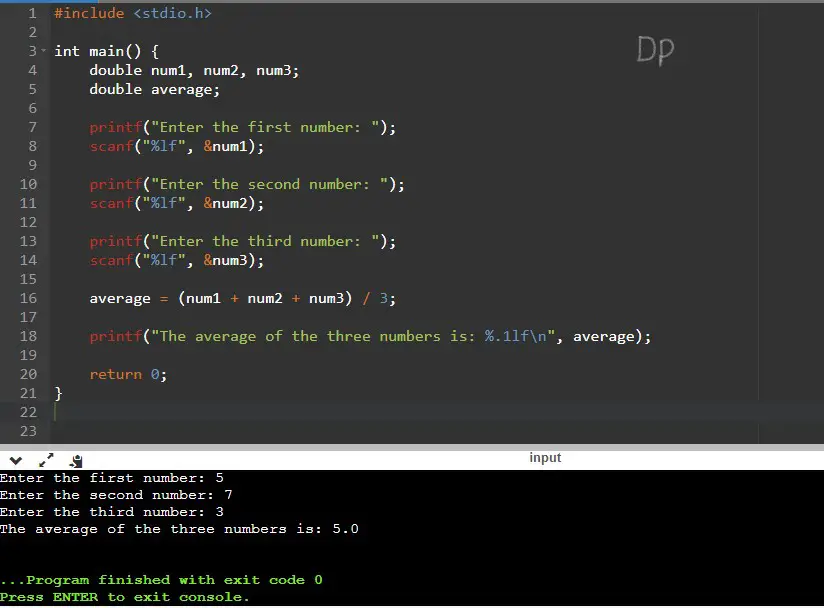