This C program finds the Highest Common Factor (HCF) of two given numbers without using recursion.
Problem statement
Write a C program to find the Highest Common Factor (HCF) of two numbers without using recursion.
Inputs:
- Two integers represent the numbers for which the HCF needs to be found.
Output:
- The HCF of the two input numbers.
Note:
- The program assumes that the input numbers are positive integers.
- The program does not handle negative numbers or floating-point numbers.
C Program to Find HCF of Two Numbers without Recursion
#include <stdio.h> int findHCF(int num1, int num2) { int hcf; int smaller = (num1 < num2) ? num1 : num2; for (int i = 1; i <= smaller; i++) { if (num1 % i == 0 && num2 % i == 0) { hcf = i; } } return hcf; } int main() { int num1, num2; printf("Enter two numbers: "); scanf("%d %d", &num1, &num2); int hcf = findHCF(num1, num2); printf("The HCF of %d and %d is %d\n", num1, num2, hcf); return 0; }
How it works
- The program starts by prompting the user to enter two numbers.
- The
scanf()
function is used to read the two input numbers from the user. - The
main()
function then calls thefindHCF()
function, passing the two input numbers as arguments. - Inside the
findHCF()
function, a variablehcf
is declared to store the highest common factor. - The function determines the smaller of the two input numbers using the ternary operator.
- A
for
loop is used to iterate from 1 up to the value of the smaller number. - For each iteration, the function checks if both input numbers are divisible by the current value of
i
. - If they are, the
hcf
variable is updated with the current value ofi
. - At the end of the loop, the
hcf
variable will contain the highest common factor of the two input numbers. - The
findHCF()
function returns the value ofhcf
to themain()
function. - Finally, the
main()
function displays the result by printing the HCF of the two input numbers.
The program uses a simple iterative approach to find the highest common factor without using recursion. By checking divisibility of the numbers starting from 1 and updating the hcf
variable whenever a common factor is found, the program determines the HCF efficiently.
Input / Output
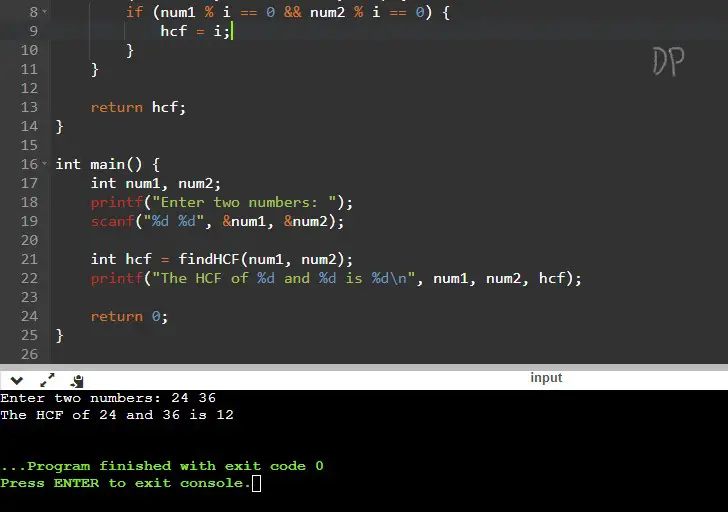