This C program checks whether a given number is a strong number or not, where the number is entered by the user. The program then determines whether the number is a strong number using a loop and prints a message to the screen.
Problem Statement
Write a C program that checks whether a given number is a strong number or not, where the number is entered by the user. The program should prompt the user to enter the number, determine whether it is a strong number using a loop, and print a message to the screen indicating whether the number is a strong number or not.
Solution
#include <stdio.h> int main() { int n, i, digit, fact, sum = 0, temp; // Get the value of n from user printf("Enter a positive integer: "); scanf("%d", &n); temp = n; // Check whether the number is a strong number or not while (temp != 0) { digit = temp % 10; fact = 1; for (i = 1; i <= digit; i++) { fact *= i; } sum += fact; temp /= 10; } // Print the appropriate message to the screen if (sum == n) printf("%d is a strong number.", n); else printf("%d is not a strong number.", n); return 0; }
Output
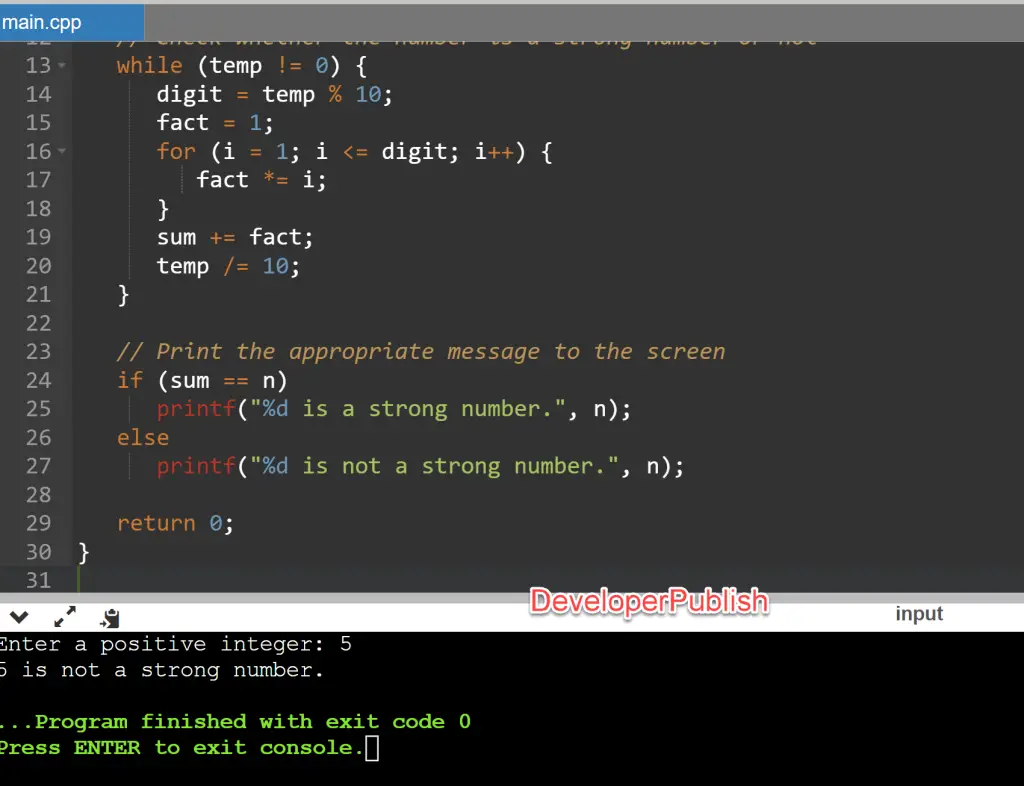
Explanation
The program starts by declaring variables to hold the value of the number entered by the user (n), the individual digits of the number (digit), the factorial of each digit (fact), the sum of the factorials (sum), and a temporary variable to store the value of n (temp).
The program then prompts the user to enter a positive integer, and stores the value in the n variable.
Next, the program checks whether the number is a strong number or not using a while loop. The loop continues until the value of temp becomes 0. In each iteration of the loop, the program extracts the last digit of the number using the modulo operator (%), calculates the factorial of the digit using a for loop, adds the factorial to the sum variable, and removes the last digit of the number using integer division (/).
After the loop, the program checks whether the sum of the factorials is equal to the value of n. If the sum is equal to n, the program prints a message saying that the number is a strong number. Otherwise, the program prints a message saying that the number is not a strong number.
Finally, the program exits.
Conclusion
This C program demonstrates how to check whether a given number is a strong number or not. It prompts the user to enter the number, determines whether it is a strong number using a loop, and prints a message to the screen indicating whether the number is a strong number or not.