This C program converts a decimal number to its equivalent octal representation.
Problem statement
You need to convert a decimal number to its octal representation.
C program to Convert Decimal to Octal
#include <stdio.h> int decimalToOctal(int decimalNumber) { int octalNumber = 0, i = 1; while (decimalNumber != 0) { octalNumber += (decimalNumber % 8) * i; decimalNumber /= 8; i *= 10; } return octalNumber; } int main() { int decimalNumber; printf("Decimal to Octal Converter\n"); printf("Enter a decimal number: "); scanf("%d", &decimalNumber); int octalNumber = decimalToOctal(decimalNumber); printf("Octal number: %d\n", octalNumber); return 0; }
How it works?
- The program defines a function
decimalToOctal()
that takes a decimal number as input and returns the equivalent octal number. - Inside the
decimalToOctal()
function, we initialize theoctalNumber
variable to 0 andi
variable to 1. These variables will be used to calculate the octal number. - We use a while loop that continues until the decimal number becomes 0.
- Inside the loop, we update the
octalNumber
by adding the remainder ofdecimalNumber
divided by 8 multiplied byi
. This is done to convert the remainder to the corresponding octal digit at the appropriate place value. - We then divide
decimalNumber
by 8 to move on to the next digit. - We multiply
i
by 10 to update the place value for the next digit. - Finally, we return the
octalNumber
. - In the
main()
function, we prompt the user to enter a decimal number and store it in thedecimalNumber
variable usingscanf()
. - We call the
decimalToOctal()
function withdecimalNumber
as an argument and store the returned octal number in theoctalNumber
variable. - Finally, we display the converted octal number using
printf()
.
Input/Output
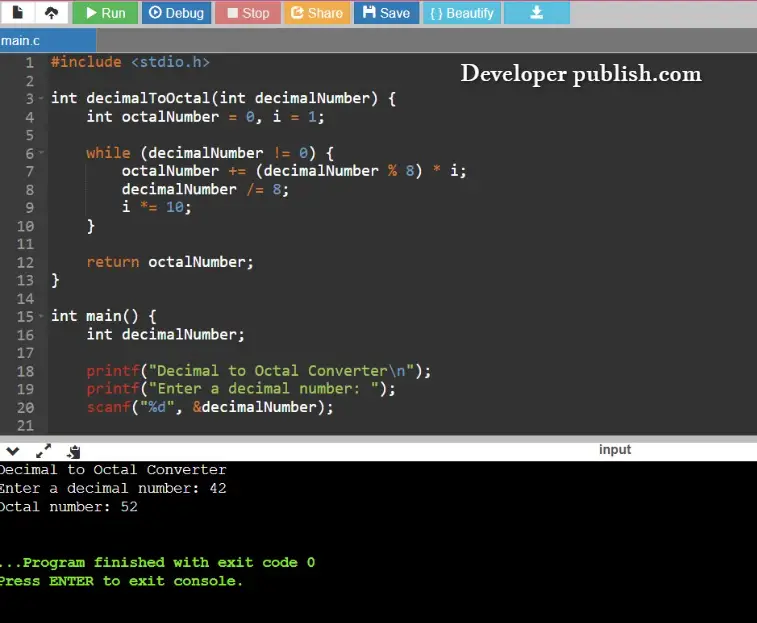