This C program finds missing numbers in an array. It takes an array as input and prints the numbers that are missing from the array. The program uses a simple nested loop approach to check each number from 1 to n and compares it with the elements of the array.
Problem statement
Given an array of integers, find and print the missing numbers in the range from 1 to n, where n is the size of the array plus 1.
C Program to Find Missing Numbers in Array
#include <stdio.h> void findMissingNumbers(int arr[], int n) { int i, j, found; printf("Missing numbers: "); // Check each number from 1 to n for (i = 1; i <= n; i++) { found = 0; // Check if the number is present in the array for (j = 0; j < n; j++) { if (arr[j] == i) { found = 1; break; } } // If the number is not found, print it if (found == 0) { printf("%d ", i); } } printf("\n"); } int main() { int arr[] = {4, 2, 1, 6, 5}; int size = sizeof(arr) / sizeof(arr[0]); printf("Array: "); for (int i = 0; i < size; i++) { printf("%d ", arr[i]); } printf("\n"); findMissingNumbers(arr, size + 1); return 0; }
How it works?
- The program initializes an array
arr
and its sizesize
. - The contents of the array are printed.
- The
findMissingNumbers
function is called with the array and its size incremented by 1. - In the
findMissingNumbers
function, a nested loop is used to iterate through numbers from 1 to n. - For each number, it checks if it is present in the array by comparing it with each element in the array.
- If a number is not found in the array, it is considered missing and printed.
- After checking all the numbers, the function returns to the
main
function. - The program terminates.
Input/Output
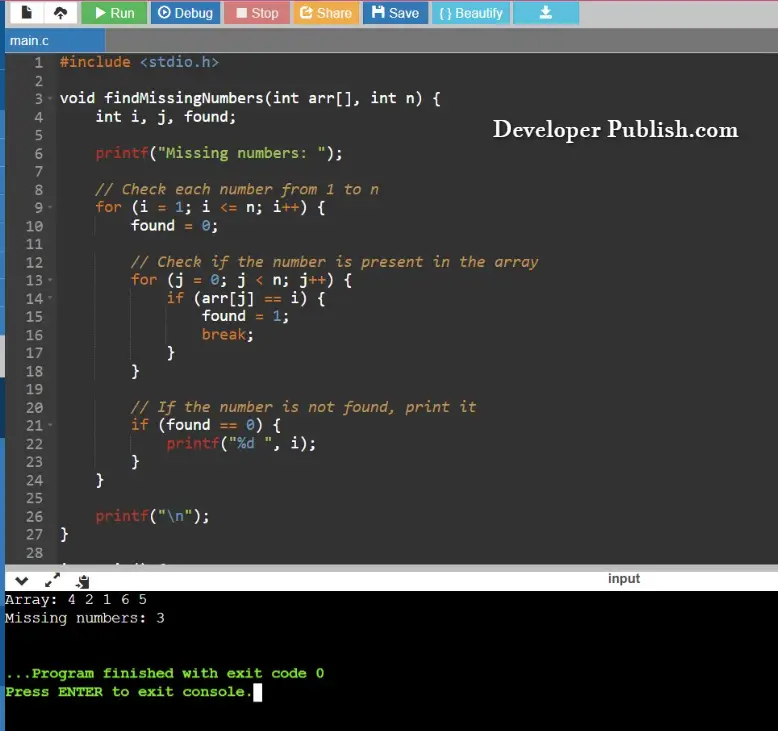