This C program finds the sum of the first N natural numbers, where N is entered by the user. The program then calculates the sum using a loop and prints it to the screen.
Problem Statement
Write a C program that finds the sum of the first N natural numbers, where N is entered by the user. The program should prompt the user to enter the value of N, calculate the sum using a loop, and print it to the screen.
Solution
#include <stdio.h> int main() { int n, i, sum = 0; // Get the value of n from user printf("Enter the value of n: "); scanf("%d", &n); // Calculate the sum using a loop for (i = 1; i <= n; i++) { sum += i; } // Print the sum to the screen printf("The sum of first %d natural numbers is %d", n, sum); return 0; }
Output
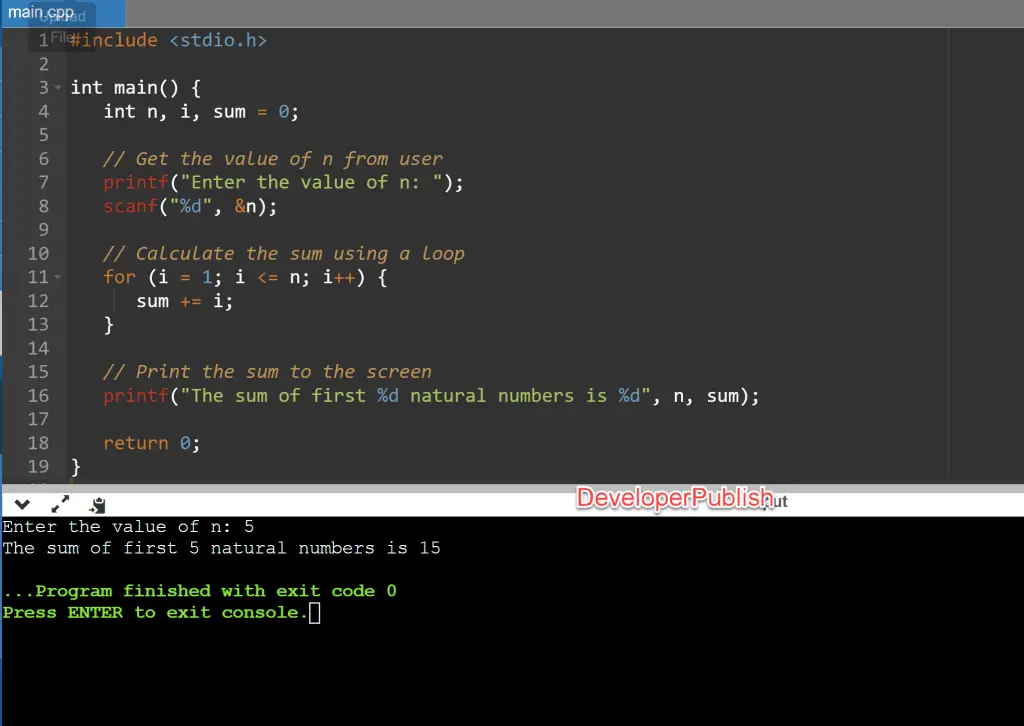
Explanation
The program starts by declaring variables to hold the value of N (n), a loop counter (i), and the sum of the first N natural numbers (sum).
The program then prompts the user to enter the value of N, and stores the value in the n variable.
Next, the program calculates the sum of the first N natural numbers using a for loop with i as the loop counter. For each iteration of the loop, the program adds i to the sum variable.
After the loop, the program prints the sum of the first N natural numbers to the screen using printf() function.
Finally, the program exits.
Conclusion
This C program demonstrates how to find the sum of the first N natural numbers. It prompts the user to enter the value of N, calculates the sum using a loop, and prints it to the screen.