This C program checks whether a given number is prime or not, where the number is entered by the user. The program then determines whether the number is prime using a loop and prints a message to the screen.
Problem Statement
Write a C program that checks whether a given number is prime or not, where the number is entered by the user. The program should prompt the user to enter the number, determine whether it is prime using a loop, and print a message to the screen indicating whether the number is prime or not.
Solution
#include <stdio.h> int main() { int n, i, flag = 0; // Get the value of n from user printf("Enter a positive integer: "); scanf("%d", &n); // Check whether the number is prime or not for (i = 2; i <= n/2; ++i) { // Condition for non-prime number if(n%i == 0) { flag = 1; break; } } // Print the appropriate message to the screen if (n == 1) { printf("1 is neither prime nor composite."); } else { if (flag == 0) printf("%d is a prime number.", n); else printf("%d is not a prime number.", n); } return 0; }
Output
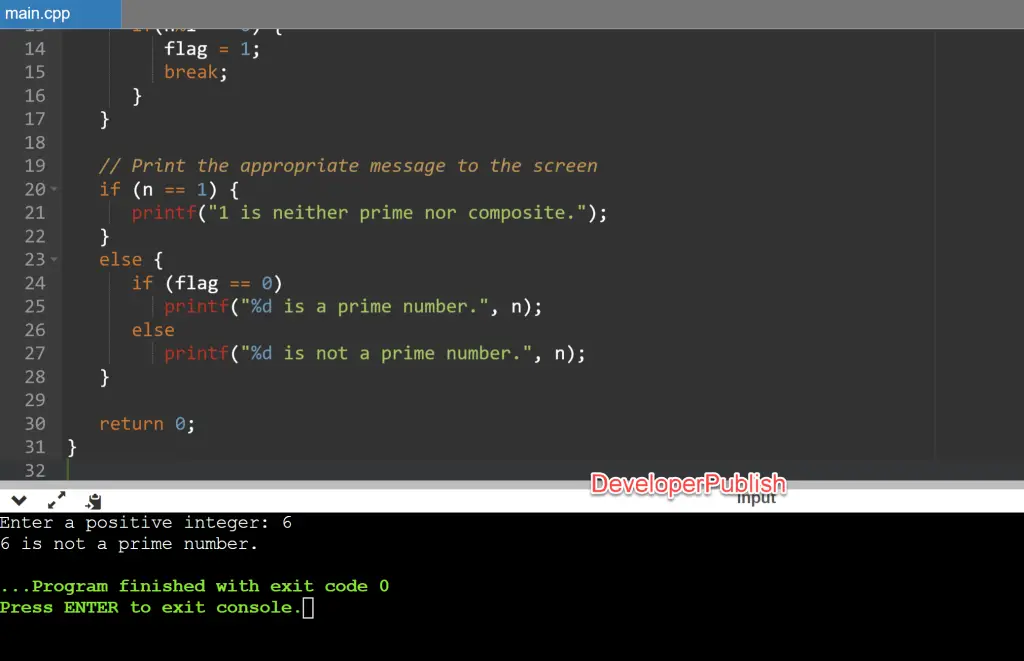
Explanation
The program starts by declaring variables to hold the value of the number entered by the user (n), a loop counter (i), and a flag variable (flag) that will be used to indicate whether the number is prime or not.
The program then prompts the user to enter a positive integer, and stores the value in the n variable.
Next, the program checks whether the number is prime or not using a for loop with i as the loop counter. The loop starts at 2 and goes up to n/2. For each iteration of the loop, the program checks whether the number is divisible by i using the modulo operator (%). If the number is divisible by i, it means that the number is not prime, and the program sets the flag variable to 1 and breaks out of the loop.
After the loop, the program prints an appropriate message to the screen depending on whether the number is prime or not. If the number is 1, the program prints a message saying that 1 is neither prime nor composite. Otherwise, if the flag variable is 0, the program prints a message saying that the number is prime. If the flag variable is 1, the program prints a message saying that the number is not prime.
Finally, the program exits.
Conclusion
This C program demonstrates how to check whether a given number is prime or not. It prompts the user to enter the number, determines whether it is prime using a loop, and prints a message to the screen indicating whether the number is prime or not.