This C program converts an octal number to its equivalent binary representation.
Converting numbers between different number systems is a common task in computer programming. The octal number system is a base-8 system that uses digits ranging from 0 to 7. On the other hand, the binary number system is a base-2 system that uses only two digits, 0 and 1.
Problem Statement
Write a C program to convert an octal number to its binary representation using a specific format. The program should follow the given format and convert each octal digit to its corresponding binary representation.
The program should have the following specifications:
- The program should prompt the user to enter an octal number.
- Read the octal number entered by the user.
- Convert each digit of the octal number to its binary representation using the following rules:
- Each octal digit is converted to a corresponding binary representation.
- The binary representation of each octal digit is determined as follows:
- Convert each octal digit to a 3-digit binary number.
- For example, octal digit 0 is converted to binary 000, octal digit 1 is converted to binary 001, octal digit 2 is converted to binary 010, and so on.
- Combine the binary representations of each octal digit to form the final binary number.
- Display the resulting binary number to the user.
- The program should be implemented using the C programming language.
Ensure that your program handles valid inputs correctly and provides the expected output.
Enter an octal number: 111
Binary number: 1001001
In this example, the user entered the octal number 111. The program converts each digit (1,1 and 1) to its binary representation (100,100 and 1, respectively). Finally, the binary representations are combined to form the binary number 1001001, which is displayed as the output.
Note:
- Octal numbers are represented using digits from 0 to 7.
- Binary numbers are represented using digits 0 and 1.
C Program to Convert Octal to Binary Number
#include <stdio.h> // Function to convert an octal digit to binary int octalToBinary(int octalDigit) { int binary = 0, base = 1; // Convert each octal digit to binary while (octalDigit > 0) { binary += (octalDigit % 10) * base; octalDigit /= 10; base *= 2; } return binary; } // Function to convert octal to binary void convertOctalToBinary(int octal) { int binary = 0, place = 1; // Convert each digit of the octal number to binary while (octal > 0) { int octalDigit = octal % 10; int binaryDigit = octalToBinary(octalDigit); binary += binaryDigit * place; place *= 1000; // Each octal digit corresponds to 3 binary digits octal /= 10; } printf("Binary number: %d\n", binary); } int main() { int octal; printf("Enter an octal number: "); scanf("%d", &octal); convertOctalToBinary(octal); return 0; }
How it Works
- The program starts by including the necessary header file
stdio.h
for input/output operations. - Two functions are defined:
octalToBinary
andconvertOctalToBinary
. TheoctalToBinary
function converts a single octal digit to its binary representation, and theconvertOctalToBinary
function converts the entire octal number to binary using theoctalToBinary
function. - In the
octalToBinary
function:- The function takes a single octal digit as input, which is represented by the variable
octalDigit
. - Inside the function, there are two variables:
binary
andbase
. Thebinary
variable stores the calculated binary representation of the octal digit, and thebase
variable represents the base value used for the conversion. - The conversion process starts with initializing
binary
andbase
to 0 and 1, respectively. - A while loop is used to convert each octal digit to binary. The loop continues as long as the
octalDigit
is greater than 0. - Inside the loop, the rightmost digit of the
octalDigit
is extracted by using the modulus operator%
with 10. This digit is then multiplied by thebase
and added to thebinary
variable. - The
octalDigit
is divided by 10 to remove the rightmost digit, and thebase
is multiplied by 2 to update the base for the next iteration. - This process continues until all the digits of the
octalDigit
have been converted to binary. - Finally, the
binary
variable, which contains the binary representation of the octal digit, is returned from the function.
- The function takes a single octal digit as input, which is represented by the variable
- In the
convertOctalToBinary
function:- The function takes an octal number as input, which is represented by the variable
octal
. - Inside the function, there are two variables:
binary
andplace
. Thebinary
variable stores the final binary representation of the octal number, and theplace
variable represents the place value used for combining binary digits. - The conversion process starts with initializing
binary
andplace
to 0 and 1, respectively. - A while loop is used to convert each digit of the octal number to binary. The loop continues as long as the
octal
number is greater than 0. - Inside the loop, the rightmost digit of the
octal
number is extracted by using the modulus operator%
with 10, and it is stored in the variableoctalDigit
. - The
octalDigit
is converted to binary by calling theoctalToBinary
function and passingoctalDigit
as the argument. The returned binary digit is stored in the variablebinaryDigit
. - The
binaryDigit
is multiplied by theplace
and added to thebinary
variable to combine the binary digits. - The
place
is multiplied by 1000 (since each octal digit corresponds to 3 binary digits) to update the place value for the next iteration. - The
octal
number is divided by 10 to remove the rightmost digit. - This process continues until all the digits of the
octal
number have been converted to binary. - Finally, the resulting
binary
number is printed to the console.
- The function takes an octal number as input, which is represented by the variable
- In the
main
function:- The function starts by declaring the variable
octal
to store the octal number entered by the user. - The user is prompted to enter an octal number using the
printf
function. - The
scanf
function is used to read the user input and store it in theoctal
variable. - The
convertOctalToBinary
function is called with theoctal
number as the argument to perform the conversion. - The resulting binary number is displayed to the user using the
printf
function.
- The function starts by declaring the variable
By following these steps, the program takes an octal number as input, converts each digit to its binary representation using the specified format, and displays the resulting binary number to the user.
Input /Output
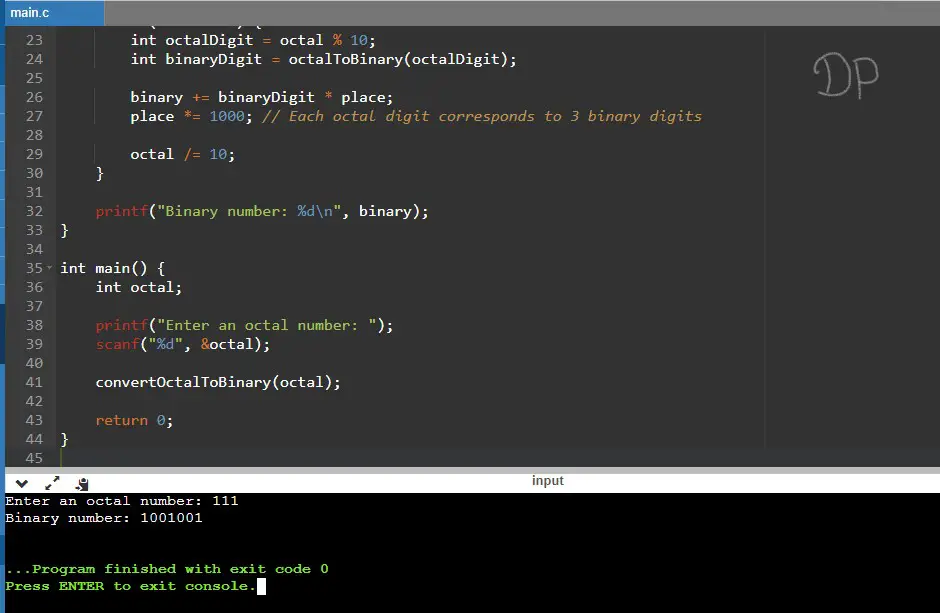