Introduction
This C program calculates the Nth Fibonacci number using recursion. The Fibonacci sequence is a series of numbers in which each number is the sum of the two preceding ones. The program uses a recursive function to calculate the Nth Fibonacci number.
Problem statement
Write a C program to find the Nth Fibonacci number using recursion.
C Program to Find Nth Fibonacci Number using Recursion
#include <stdio.h> int fibonacci(int n) { if (n <= 1) return n; else return fibonacci(n - 1) + fibonacci(n - 2); } int main() { int n; printf("Enter the value of N: "); scanf("%d", &n); int result = fibonacci(n); printf("The %dth Fibonacci number is: %d\n", n, result); return 0; }
How it works
- The program defines a function
fibonacci()
that takes an integern
as a parameter and returns the Nth Fibonacci number. - In the
fibonacci()
function, there is a base case where ifn
is less than or equal to 1, it returnsn
itself (0 for n=0 and 1 for n=1). - For values of
n
greater than 1, the function recursively calls itself withn-1
andn-2
, and adds the results to get the Fibonacci number. - In the
main()
function, the user is prompted to enter the value ofN
. - The
scanf()
function is used to read the input value from the user and store it in the variablen
. - The
fibonacci()
function is called with the input valuen
to calculate the Nth Fibonacci number. - The result is then printed on the console.
Input/Output
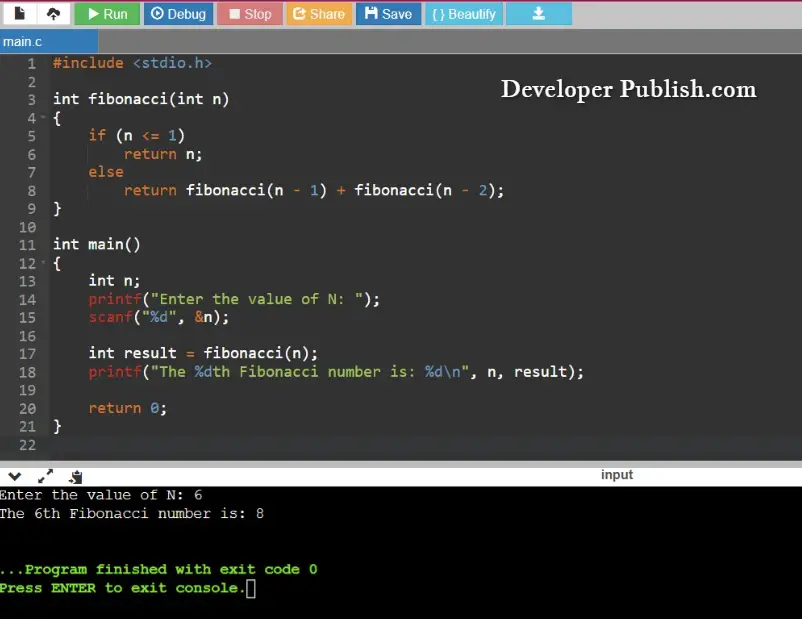