Swapping two numbers is a common task in programming. In Python, it can be achieved using a temporary variable. However, an alternative method allows swapping two numbers without using an extra variable. In this program, we will explore this technique and implement it in Python.
Problem statement
Write a Python program that takes two numbers as input and swaps their values without using a third variable.
Python Program to Swap Two Numbers without using Third Variable
# Function to swap two numbers without using a third variable def swap_numbers(a, b): print("Before swapping:") print("a =", a) print("b =", b) a = a + b b = a - b a = a - b print("After swapping:") print("a =", a) print("b =", b) # Main program num1 = float(input("Enter the first number: ")) num2 = float(input("Enter the second number: ")) swap_numbers(num1, num2)
Input\output
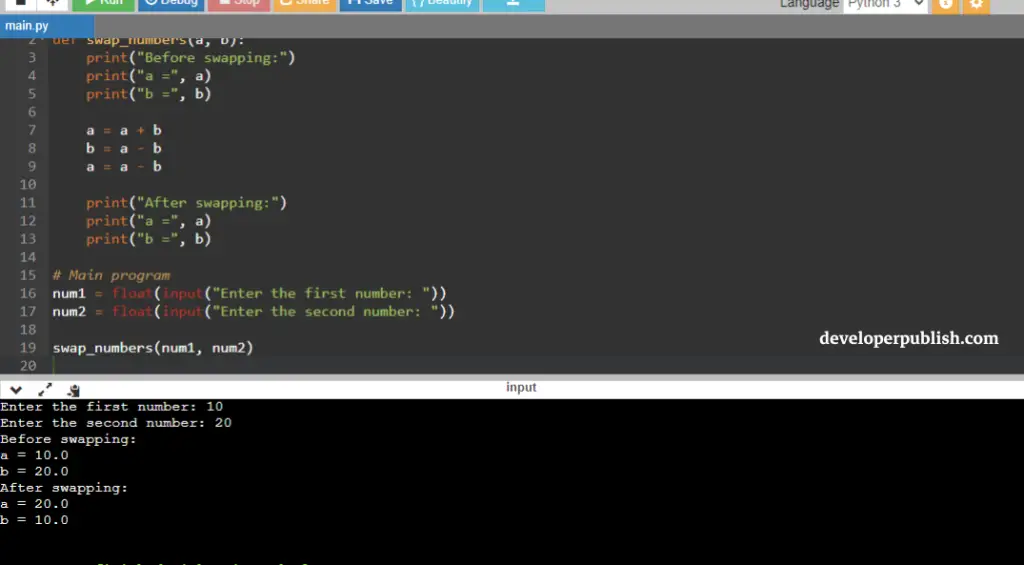