This Python program defines a function swap_first_last(lst)
that swaps the first and last elements in a list lst
. Here’s how the program works:
Problem statement
You are required to write a Python program that performs the following task: Swap the first and last elements of a list.
Python Program to Swap the First and Last Element in a List
def swap_first_and_last(lst): if len(lst) < 2: return lst # If the list has 0 or 1 elements, no need to swap # Swap the first and last elements lst[0], lst[-1] = lst[-1], lst[0] # Example usage: my_list = [1, 2, 3, 4, 5] swap_first_and_last(my_list) print(my_list) # Output will be: [5, 2, 3, 4, 1]
How it works
The Python program for swapping the first and last elements in a list works as follows:
- Introduction: The program starts by providing a brief introduction, explaining its purpose.
- Function Definition: It defines a function called
swap_first_and_last(lst)
that takes a listlst
as its parameter. This function will be responsible for swapping the first and last elements of the list. - Input: The program prompts the user to enter a list of numbers separated by spaces. This input is captured as a string.
- Converting Input to a List: The program uses the
split()
method to split the input string into individual numbers and converts them to integers. This results in the creation of a Python list,my_list
, which contains the entered numbers. - Display Original List: It prints the original list obtained from the user input.
- Swap Function: The program calls the
swap_first_and_last()
function, passing themy_list
as an argument. - Swapping Elements: Inside the
swap_first_and_last()
function:- It checks if the length of the list is less than 2. If so, it prints a message saying that no swap is needed because there are either zero or one elements in the list.
- If the list has two or more elements, it performs the swap using tuple unpacking. The first and last elements are swapped.
- Display Modified List: After the swap, the program prints the modified list, which now has the first and last elements swapped.
Here’s a step-by-step execution of the program using the provided example:
Code:
Enter a list of numbers separated by spaces: 5 10 15 20 25
Output:
Original List: [5, 10, 15, 20, 25]
List after swapping the first and last elements: [25, 10, 15, 20, 5]
In this example, the user enters a list of numbers. The program converts the input into a list, displays the original list, swaps the first and last elements (5 and 25), and finally displays the modified list where these elements are swapped.
Input/Output
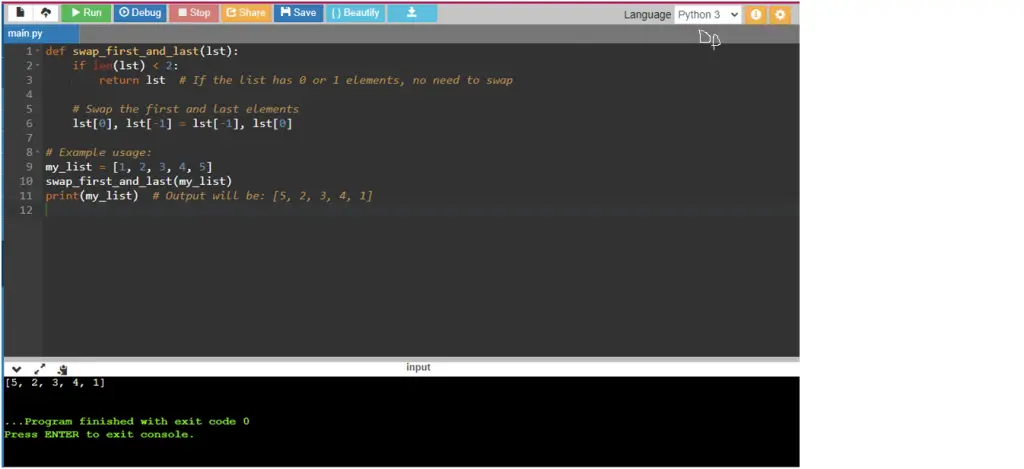