A linked list is a linear data structure used in computer science to store and manage collections of elements. In this Python program, we tackle the problem of reversing the order of the first N elements in a given linked list while leaving the rest of the list unchanged.
Problem Statement
Given a linked list and a positive integer N, reverse the order of the first N elements in the list, while keeping the remaining elements unchanged.
Python Program to Reverse First N Elements of a Linked List
class Node: def __init__(self, data): self.data = data self.next = None class LinkedList: def __init__(self): self.head = None def append(self, data): new_node = Node(data) if not self.head: self.head = new_node else: current = self.head while current.next: current = current.next current.next = new_node def reverse_first_n(self, n): prev = None current = self.head for _ in range(n): if current: next_node = current.next current.next = prev prev = current current = next_node self.head = prev def display(self): current = self.head while current: print(current.data, end=" -> ") current = current.next print("None") # Input: Creating a linked list linked_list = LinkedList() elements = [1, 2, 3, 4, 5, 6, 7, 8, 9] for element in elements: linked_list.append(element) n = 4 # Number of elements to reverse print("Original Linked List:") linked_list.display() linked_list.reverse_first_n(n) print("\nLinked List after Reversing First", n, "Elements:") linked_list.display()
Input / Output
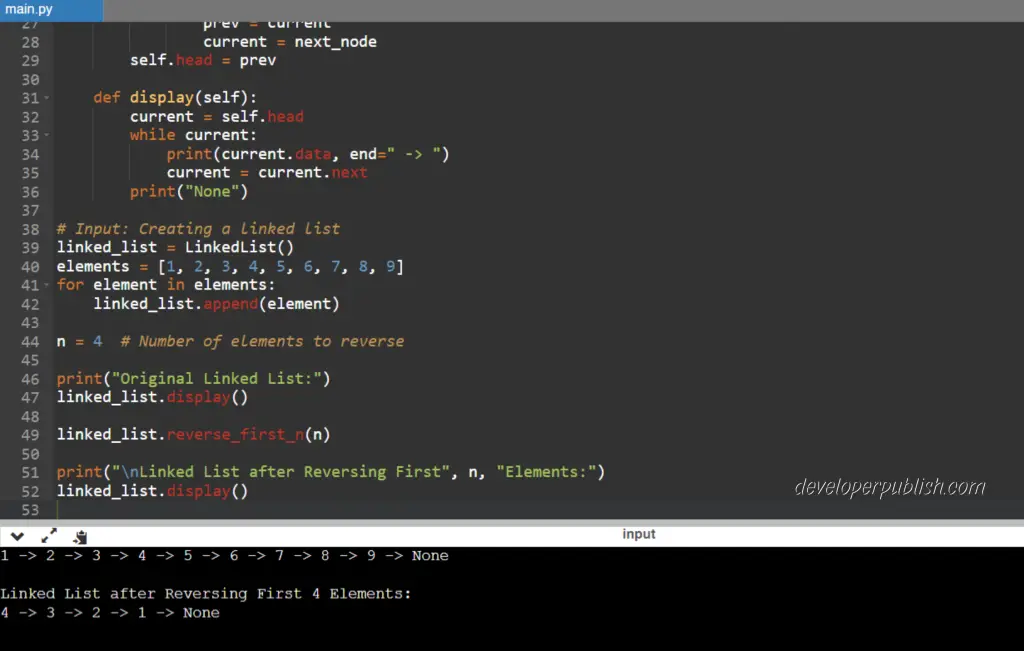