In this Python program, we will create a singly linked list and remove duplicate elements from it. A linked list is a data structure consisting of nodes, where each node contains a value and a reference (or link) to the next node in the list.
Problem Statement
Given a linked list, remove any duplicate elements so that only the distinct elements remain in the list.
Python Program to Remove Duplicates from a Linked List
# Introduction # In this Python program, we will create a singly linked list and remove duplicate elements from it. A linked list is a data structure consisting of nodes, where each node contains a value and a reference (or link) to the next node in the list. # Problem Statement # Given a linked list, remove any duplicate elements so that only the distinct elements remain in the list. # Program class Node: def __init__(self, data): self.data = data self.next = None class LinkedList: def __init__(self): self.head = None def append(self, data): new_node = Node(data) if self.head is None: self.head = new_node return current = self.head while current.next: current = current.next current.next = new_node def display(self): current = self.head while current: print(current.data, end=' ') current = current.next print() def remove_duplicates(self): current = self.head while current: runner = current while runner.next: if runner.next.data == current.data: runner.next = runner.next.next else: runner = runner.next current = current.next # How it Works # 1. We define two classes, Node and LinkedList. Node represents an element in the linked list, and LinkedList is used to manage the list. # 2. The `append` method is used to add elements to the linked list. # 3. The `display` method is used to print the elements of the linked list. # 4. The `remove_duplicates` method is used to remove duplicate elements from the linked list. # 5. We iterate through the list, and for each element, we iterate through the rest of the list to remove any duplicates. # Input/Output if __name__ == "__main__": linked_list = LinkedList() elements = [1, 2, 2, 3, 4, 4, 5] for element in elements: linked_list.append(element) print("Original Linked List:") linked_list.display() linked_list.remove_duplicates() print("\nLinked List after removing duplicates:") linked_list.display()
How it works
- We define two classes, Node and LinkedList.
- Node represents an element in the linked list, and LinkedList is used to manage the list.
- The `append` method is used to add elements to the linked list.
- The `display` method is used to print the elements of the linked list.
- The `remove_duplicates` method is used to remove duplicate elements from the linked list.
- We iterate through the list, and for each element, we iterate through the rest of the list to remove any duplicates.
Input / Output
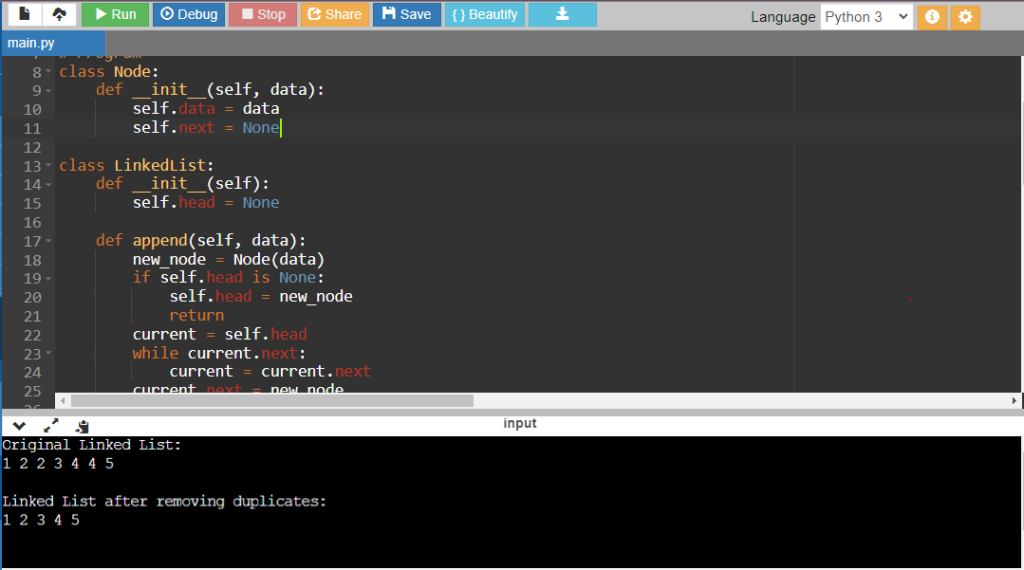