The python program demonstrates how to print the boundary traversal of a binary tree, which includes printing the left boundary, leaves, and right boundary nodes in a counter-clockwise order.
Problem Statement
Given a binary tree, print its boundary traversal in a counter-clockwise order in python.
Python Program to Print Boundary Traversal of Binary Tree
class TreeNode: def __init__(self, val): self.val = val self.left = None self.right = None def print_left_boundary(root): if root: if root.left: print(root.val, end=" ") print_left_boundary(root.left) elif root.right: print(root.val, end=" ") print_left_boundary(root.right) def print_leaves(root): if root: print_leaves(root.left) if not root.left and not root.right: print(root.val, end=" ") print_leaves(root.right) def print_right_boundary(root): if root: if root.right: print_right_boundary(root.right) print(root.val, end=" ") elif root.left: print_right_boundary(root.left) print(root.val, end=" ") def print_boundary_traversal(root): if root: print(root.val, end=" ") print_left_boundary(root.left) print_leaves(root) print_right_boundary(root.right) # Example Binary Tree root = TreeNode(1) root.left = TreeNode(2) root.left.left = TreeNode(3) root.left.right = TreeNode(4) root.right = TreeNode(5) root.right.left = TreeNode(6) root.right.right = TreeNode(7) print("Boundary Traversal:") print_boundary_traversal(root)
How it Works
The program defines a TreeNode
class to represent nodes in the binary tree. It then defines three functions to print the left boundary, leaves, and right boundary nodes separately. Finally, the print_boundary_traversal
function combines these three functions to print the complete boundary traversal of the binary tree in the required order.
Input/Output
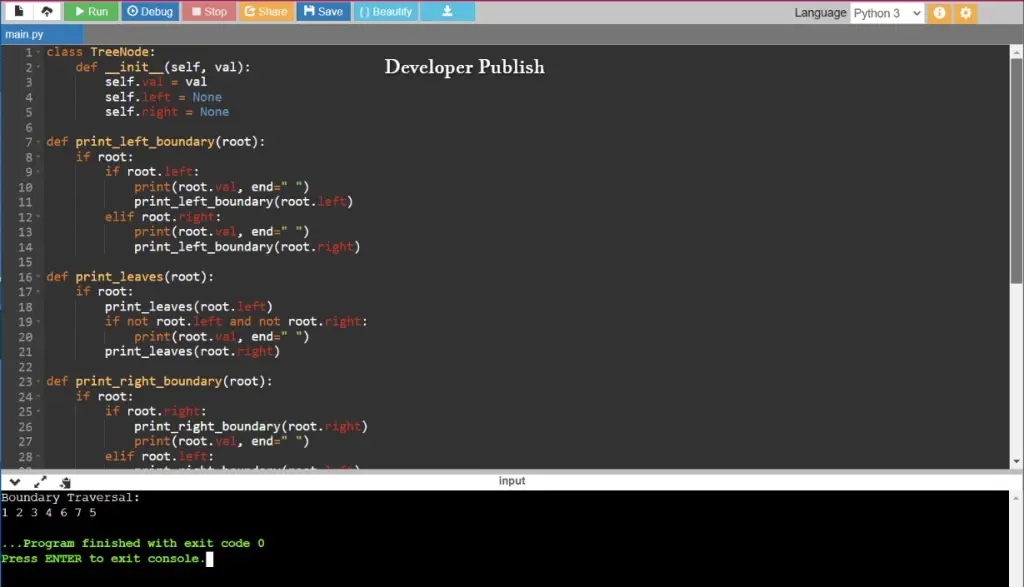