In this Python program, we will create a recursive function to print the binary equivalent of an integer. Recursion is a technique where a function calls itself to solve a smaller version of the problem. By applying recursion, we can break down the conversion of an integer to binary into smaller steps until we reach the base case.
Problem Statement
Write a Python program to print the binary equivalent of an integer using recursion.
Python Program to Print Binary Equivalent of an Integer using Recursion
def decimal_to_binary(n): if n > 1: decimal_to_binary(n // 2) print(n % 2, end='') # Getting input from the user num = int(input("Enter a decimal number: ")) # Printing the binary equivalent print("Binary equivalent of", num, "is:", end=' ') decimal_to_binary(num)
How it works
- We define a recursive function
decimal_to_binary
that takes an integern
as input. - Inside the function, we check if
n
is greater than 1. If it is, we recursively call thedecimal_to_binary
function withn // 2
. - This recursive call helps us to divide the number by 2 repeatedly until we reach the base case, which is when
n
becomes 1. - After the recursive call, we print the remainder of
n
divided by 2. This gives us the binary digit of the current step. - By using the
end
parameter of theprint
function and setting it to an empty string, we ensure that the binary digits are printed side by side without any line breaks. - Finally, we prompt the user to enter a decimal number, convert it to an integer using
int(input())
, and call thedecimal_to_binary
function with the input number.
Input / Output
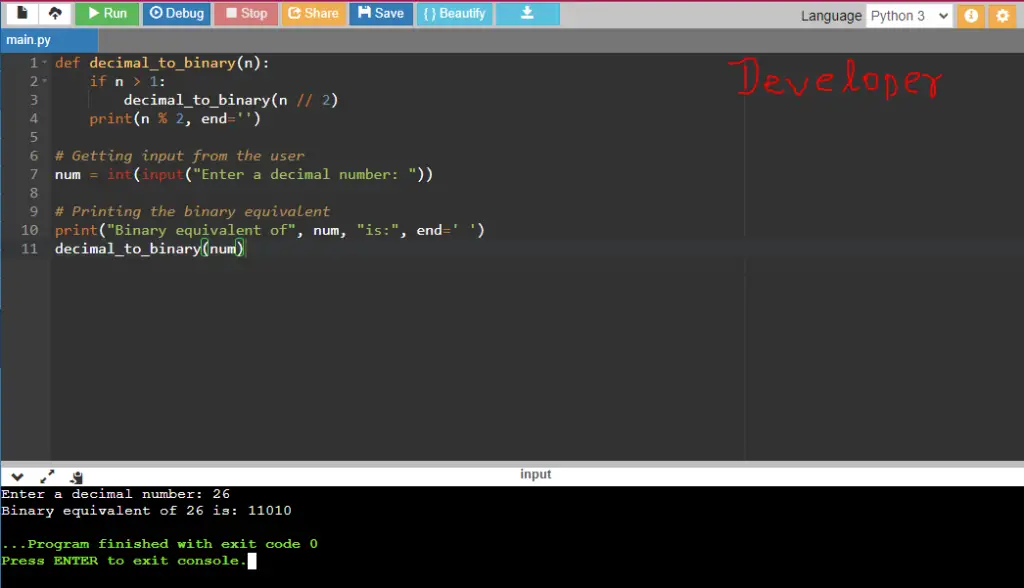