A circular doubly linked list is an advanced version of a linked list where each node contains pointers to both the next and previous nodes. This Python program demonstrates the implementation and various operations on a circular doubly linked list using Python.
Problem Statement
Given the concept of a circular doubly linked list, your task is to implement the necessary operations to create, append elements, and display the circular doubly linked list.
Python Program to Implement Circular Doubly Linked List
class Node: def __init__(self, data): self.data = data self.next = None self.prev = None class CircularDoublyLinkedList: def __init__(self): self.head = None def append(self, data): new_node = Node(data) if not self.head: self.head = new_node new_node.next = self.head new_node.prev = self.head else: current = self.head.prev current.next = new_node new_node.prev = current new_node.next = self.head self.head.prev = new_node def display(self): if not self.head: print("List is empty") return current = self.head while True: print(current.data, end=" <-> ") current = current.next if current == self.head: break print("...") # Input: Creating a circular doubly linked list circular_list = CircularDoublyLinkedList() elements = [1, 2, 3, 4, 5] for element in elements: circular_list.append(element) print("Circular Doubly Linked List:") circular_list.display()
Input / Output
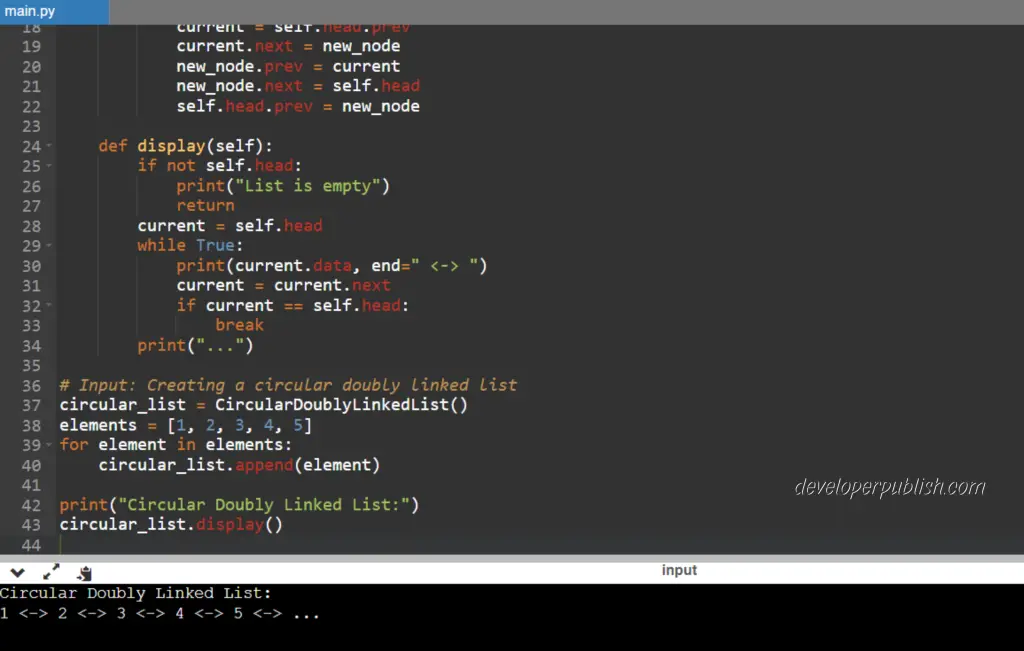