You can determine whether a number is a power of two by checking if it has only one bit set in its binary representation. If only one bit is set, then it’s a power of two. Here’s a Python program to do this with an introduction:
Program Statement
Write a Python program that checks whether a given number is a power of two. The program should prompt the user to enter a number, and then it should determine if the entered number is a power of two or not. If it is a power of two, the program should display a message indicating that the number is a power of two. Otherwise, it should display a message indicating that the number is not a power of two.
Otherwise, it should display a message indicating that the number is not a power of two. The program should continue to prompt the user for numbers until they choose to exit.
The program should have the following features:
- Define a function called
is_power_of_two
that takes an integern
as input and returnsTrue
ifn
is a power of two, andFalse
otherwise. - Inside the
is_power_of_two
function, use a loop to repeatedly dividen
by 2 until it becomes 1, checking for divisibility by 2 in each iteration. - Prompt the user to enter a number.
- Call the
is_power_of_two
function with the entered number and display an appropriate message indicating whether it is a power of two or not. - Provide an option for the user to exit the program.
That’s the program statement. You can use it as a guideline to implement the Python program to determine whether a number is a power of two.
Python Program to Find Whether a Number is a Power of Two
def is_power_of_two(n): # Check if n is positive and non-zero if n <= 0: return False # Keep dividing n by 2 until it becomes 1 while n > 1: # Check if n is divisible by 2 if n % 2 != 0: return False n = n // 2 return True # Test the function number = int(input("Enter a number: ")) if is_power_of_two(number): print(number, "is a power of two.") else: print(number, "is not a power of two.")
How it works
Here’s a step-by-step explanation of how the Python program works:
- The program defines a function called
is_power_of_two
that takes an integern
as input and returnsTrue
ifn
is a power of two, andFalse
otherwise. - Inside the
is_power_of_two
function, it first checks if the numbern
is less than or equal to 0. If it is, it immediately returnsFalse
, as powers of two are always positive. - The function enters a loop that continues until
n
becomes 1. This loop is responsible for checking the divisibility ofn
by 2. - Inside the loop, it checks if
n
is divisible by 2 by using the modulo operator (n % 2 != 0
). Ifn
is not divisible by 2 (i.e., the remainder is not 0), it meansn
is not a power of two, so the function returnsFalse
. - If
n
is divisible by 2, it updates the value ofn
by dividing it by 2 using the floor division operator (n = n // 2
). This process continues untiln
becomes 1. - Once the loop ends, it means
n
has become 1, and the function returnsTrue
to indicate that the number is a power of two. - The main part of the program prompts the user to enter a number.
- The program calls the
is_power_of_two
function with the entered number as an argument. - It checks the return value of the function. If it is
True
, the program displays a message indicating that the number is a power of two. If it isFalse
, it displays a message indicating that the number is not a power of two. - After displaying the result, the program gives an option for the user to exit or continue entering numbers.
- If the user chooses to continue, the program repeats the steps of prompting, calling the function, and displaying the result.
That’s how the program works! It repeatedly checks whether the entered numbers are powers of two and provides the corresponding output until the user decides to exit.
Input/Output
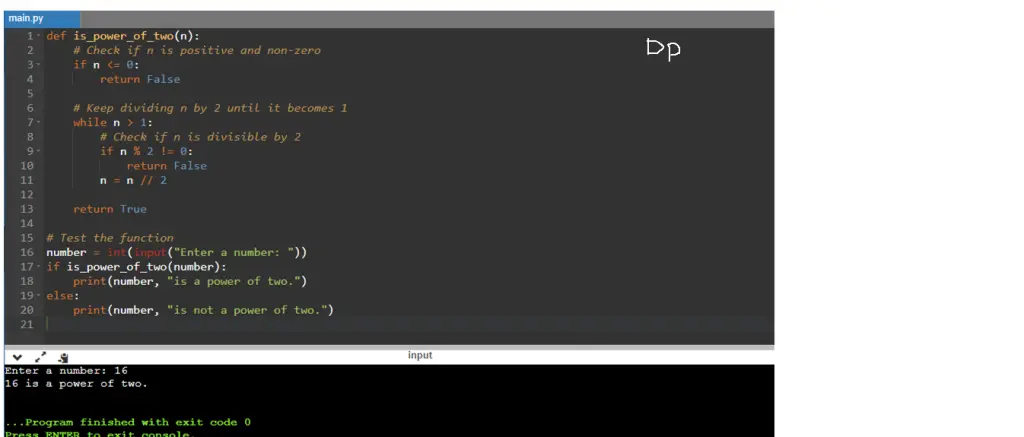