In this Python program, we’ll create a recursive function to determine how many times a given letter occurs in a string.
Problem Statement:
You need to write a Python program that calculates the frequency of a given letter in a given string using a recursive approach.
Python Program to Determine How Many Times a Given Letter Occurs in a String Recursively
def count_letter_recursive(word, letter): if len(word) == 0: return 0 elif word[0] == letter: return 1 + count_letter_recursive(word[1:], letter) else: return count_letter_recursive(word[1:], letter) # Input input_word = input("Enter a word: ") input_letter = input("Enter the letter to count: ") # Count the occurrences result = count_letter_recursive(input_word, input_letter) print(f"The letter '{input_letter}' occurs {result} times in the word '{input_word}'."
How it Works:
The program defines a recursive function count_letter_recursive that takes a word and a letter as arguments. It uses the base case of an empty word, returning 0, and two recursive cases: one where the first letter matches the given letter, incrementing the count and calling the function with the rest of the word, and another where the first letter doesn’t match, and only the function with the rest of the word is called.
Input/Output:
Enter a word: Banana
Enter the letter to count: a
The letter ‘a’ occurs 3 times in the word ‘Banana’.
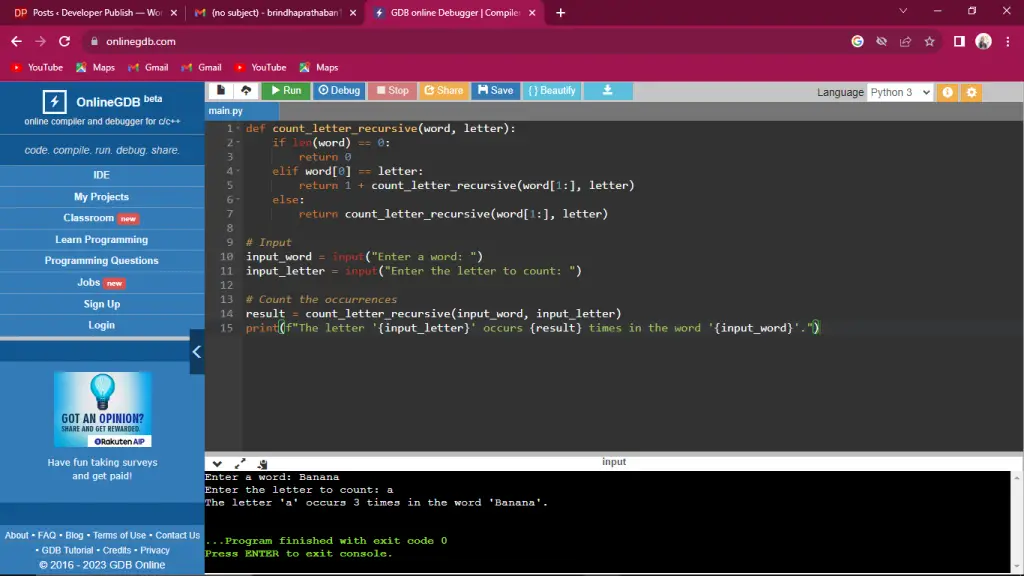