In this example, we’ll create a C# program to calculate and display the factorial of a given number, showcasing the language’s capabilities in handling mathematical operations and loops.
Problem Statement
Write a C# program to compute and print the factorial of a given non-negative integer. The program should take an input number and calculate its factorial, which is the product of all positive integers from 1 to the given number.
C# Program to Print the Factorial of a Given Number
using System; class Program { static void Main() { Console.Write("Enter a non-negative integer: "); int number = Convert.ToInt32(Console.ReadLine()); if (number < 0) { Console.WriteLine("Factorial is not defined for negative numbers."); } else { long factorial = CalculateFactorial(number); Console.WriteLine($"The factorial of {number} is: {factorial}"); } } static long CalculateFactorial(int num) { if (num == 0 || num == 1) { return 1; } else { long result = 1; for (int i = 2; i <= num; i++) { result *= i; } return result; } } }
Input / Output
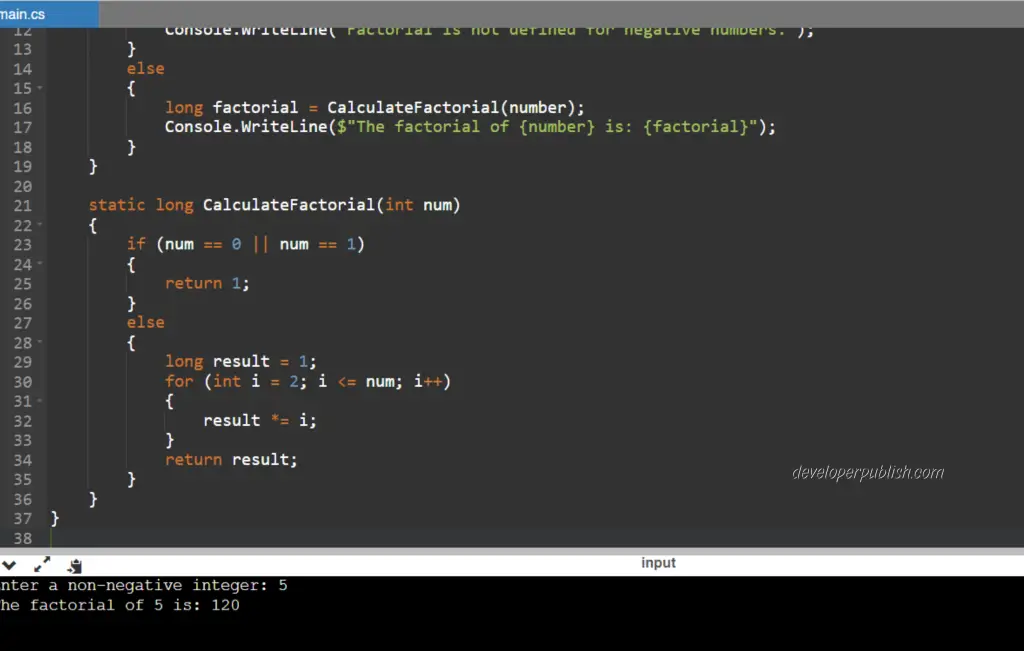