ASCII (American Standard Code for Information Interchange) is a character encoding standard that represents characters using numeric codes. Each character is assigned a unique numerical value, ranging from 0 to 127, and these values can be used to represent characters in various systems and programming languages. In this C program we look at how to read a string from the user and prints the ASCII value of each character in the string.
Problem statement
Write a C program that reads a string from the user and prints the ASCII value of each character in the string.
C Program to Print Ascii Value of All Characters in the String
#include <stdio.h> int main() { char str[100]; int i; printf("Enter a string: "); fgets(str, sizeof(str), stdin); printf("ASCII values of characters in the string:\n"); for (i = 0; str[i] != '\0'; i++) { printf("%c: %d\n", str[i], str[i]); } return 0; }
How it Works?
- The program begins by including the necessary header file
stdio.h
, which provides input/output functions such asprintf()
andfgets()
. - The
main()
function is declared, which is the entry point of the program. - A character array
str
of size 100 is declared to store the user input string. This array will hold the characters of the string along with an additional space for the null character ('\0'
) at the end. - The program prompts the user to enter a string using
printf()
. - The
fgets()
function is used to read the string from the user. It takes three arguments: the destination variable (str
), the maximum number of characters to read (size ofstr
), and the input stream (stdin
for standard input). - The program then prints a header indicating that the following output will display the ASCII values of the characters in the string.
- The
for
loop is used to iterate through each character in the string. The loop initialization setsi
to 0, and the loop condition checks if the current characterstr[i]
is not equal to the null character ('\0'
), indicating the end of the string. - Inside the loop,
printf()
is used to print the characterstr[i]
and its corresponding ASCII valuestr[i]
using the%c
and%d
format specifiers, respectively. - After printing the ASCII value of the last character, the loop increments
i
, and the process repeats until all characters in the string have been processed. - Finally, the program returns 0 to indicate successful program execution.
When you run the program, it prompts you to enter a string. Once you provide the input, it iterates through each character in the string and prints the character along with its ASCII value. This allows you to see the ASCII values of all characters in the given string.
Input / Output
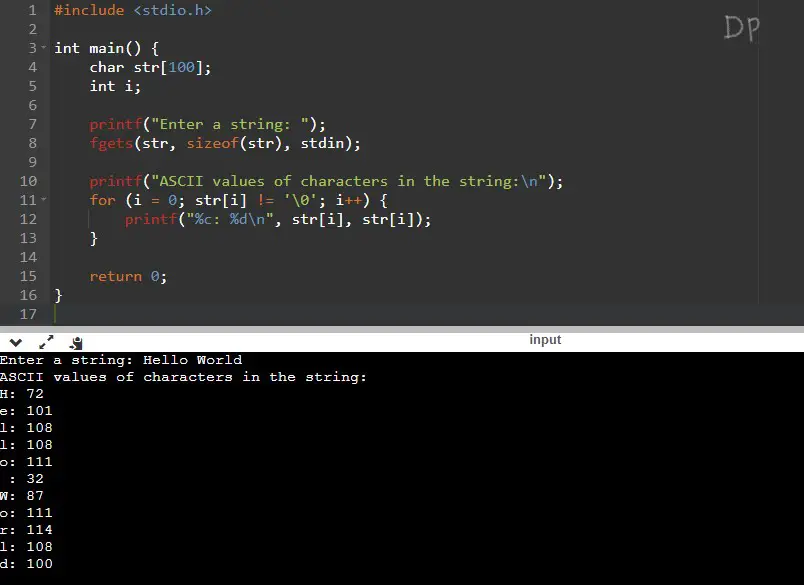
In this example, the user enters the string “Hello, World!”. The program then prints the ASCII value of each character in the string, along with the character itself. As you can see, each character is followed by a colon (:
) and its corresponding ASCII value.