This C program calculates the sum of the digits of a given positive integer using recursion. It repeatedly divides the number by 10 to extract the rightmost digit, adds it to the sum, and calls the function recursively with the remaining digits until the number becomes 0
PROGRAM STATEMENT
Given a positive integer, we need to find the sum of its digits
C Program to Find Sum of Digits of a Number using Recursion
#include <stdio.h> int sumOfDigits(int number); int main() { int number; printf("Enter a positive integer: "); scanf("%d", &number); int sum = sumOfDigits(number); printf("Sum of digits of %d = %d\n", number, sum); return 0; } int sumOfDigits(int number) { if (number == 0) { return 0; } else { return (number % 10) + sumOfDigits(number / 10);
HOW IT WORKS
- The user is prompted to enter a positive integer.
- The
sumOfDigits
function is called with the entered number as an argument. - The
sumOfDigits
function checks if the number is 0. If it is, it returns 0, indicating the base case of the recursion. - If the number is not 0, it calculates the remainder of the number divided by 10 using the modulus operator
%
. This gives us the rightmost digit of the number. - The rightmost digit is added to the sum, and the
sumOfDigits
function is called recursively with the remaining digits (number divided by 10). - Steps 3-5 are repeated until the number becomes 0.
- Once the number becomes 0, the recursion stops, and the final sum is returned.
- The main function displays the calculated sum on the screen.
INPUT/OUTPUT
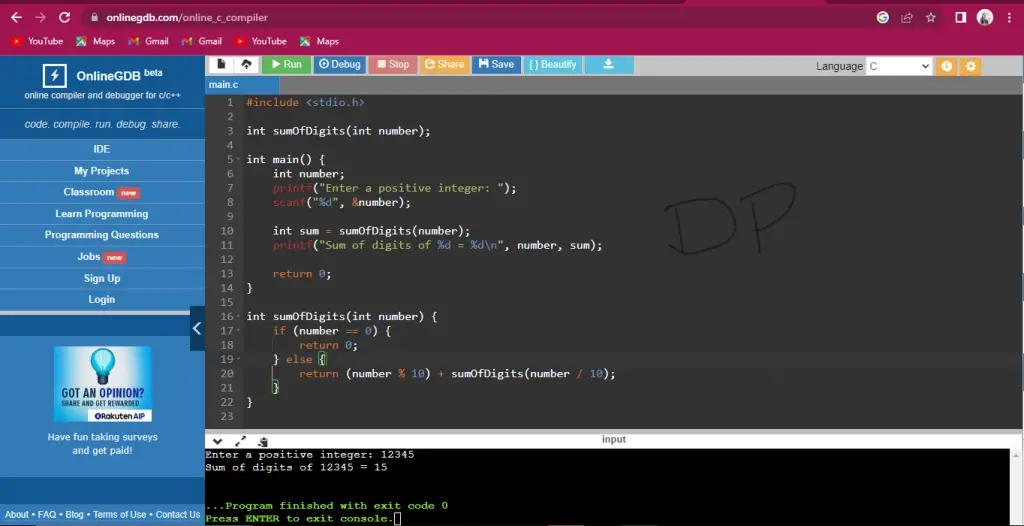