This C# program demonstrates how to find the product of two numbers using recursion. Recursion is a technique where a function calls itself to solve a problem. In this program, we will take two integer inputs from the user and find their product using a recursive function.
Problem statement
Write a C# program that calculates the product of two numbers using recursion. The program should prompt the user to enter two integers, and then it should use a recursive function to find their product. If either of the input numbers is 0, the program should return 0 as the product.
C# Program to Find Product of Two Numbers using Recursion
using System; class Program { static void Main() { Console.WriteLine("Welcome to the Product of Two Numbers Program!"); Console.WriteLine("============================================="); // Input Console.Write("Enter the first number: "); int num1 = Convert.ToInt32(Console.ReadLine()); Console.Write("Enter the second number: "); int num2 = Convert.ToInt32(Console.ReadLine()); // Calculate the product using recursion int result = MultiplyRecursive(num1, num2); // Output Console.WriteLine($"The product of {num1} and {num2} is: {result}"); } // Recursive function to find the product of two numbers static int MultiplyRecursive(int num1, int num2) { // Base case: If either number is 0, the product is 0 if (num1 == 0 || num2 == 0) return 0; // Recursive case: Multiply num1 by (num2-1) and add num1 return num1 + MultiplyRecursive(num1, num2 - 1); } }
How it works
- We start by taking two integer inputs,
num1
andnum2
, from the user. - The
MultiplyRecursive
function is called with these two numbers to find their product using recursion. - Inside the
MultiplyRecursive
function, we have two cases:- Base case: If either
num1
ornum2
is 0, we return 0 because the product of any number and 0 is 0. - Recursive case: We recursively call
MultiplyRecursive
withnum1
andnum2 - 1
and addnum1
to the result. This effectively simulates multiplication by repeatedly addingnum1
to itselfnum2
times.
- Base case: If either
- The result is displayed as the product of the two input numbers.
Input / Output
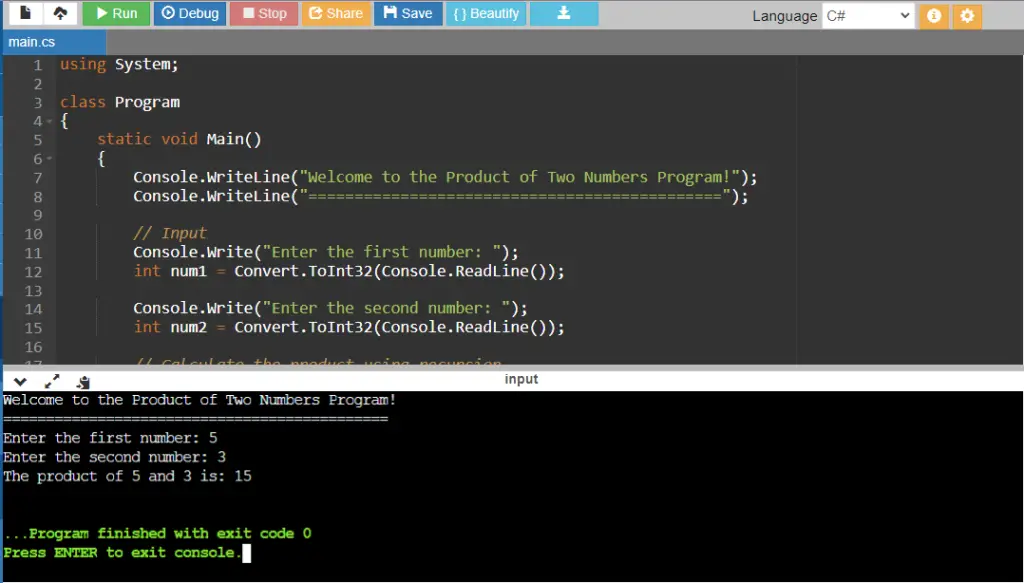