In this program, we will write a C program to find the Nth Armstrong number.
Problem Statement:
We need to take an input integer n
from the user, which denotes the position of the Armstrong number to be found. Then, we have to calculate the Nth Armstrong number and display it on the screen.
Solution:
An Armstrong number is a number that is equal to the sum of its own digits raised to the power of the number of digits. For example, 153 is an Armstrong number because 1^3 + 5^3 + 3^3 = 153. Similarly, 371 is also an Armstrong number because 3^3 + 7^3 + 1^3 = 371. Armstrong numbers are named after Michael F. Armstrong, who was a mathematician. These numbers are also known as narcissistic numbers, pluperfect digital invariants (PPDI), and sometimes also called plus perfect numbers.
#include <stdio.h> #include <math.h> int isArmstrong(int num); int main() { int n, i, count = 0; // Get the value of n from the user printf("Enter the value of n: "); scanf("%d", &n); // Find the Nth Armstrong number for (i = 0; count < n; i++) { if (isArmstrong(i)) { count++; } } printf("The %dth Armstrong number is %d", n, i - 1); return 0; } // Function to check whether a number is Armstrong or not int isArmstrong(int num) { int originalNum, remainder, n = 0, result = 0; originalNum = num; // Calculate the number of digits in the number while (originalNum != 0) { originalNum /= 10; ++n; } originalNum = num; // Calculate the sum of nth power of each digit while (originalNum != 0) { remainder = originalNum % 10; result += pow(remainder, n); originalNum /= 10; } // Check whether the number is Armstrong or not if (result == num) { return 1; } else { return 0; } }
Output
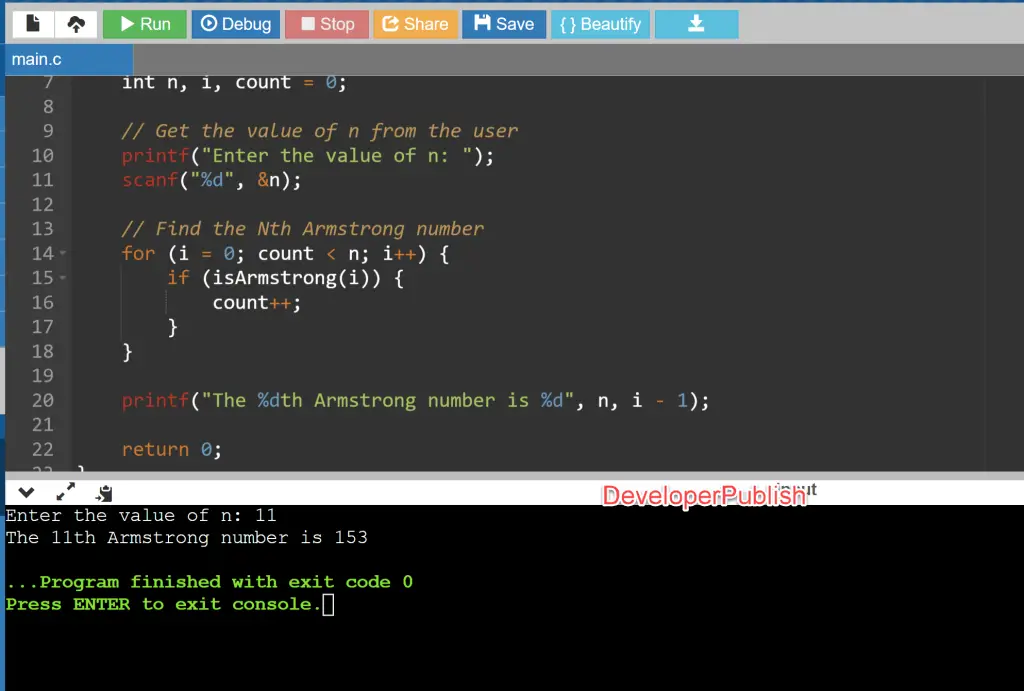
Explanation:
- We first include the standard input-output library
stdio.h
and themath.h
library for using thepow
function in the program using the#include
preprocessor directive. - Then, we declare the main function using the
int main()
syntax. - We declare three variables
n
,i
, andcount
as integers. - We use the
printf
function to prompt the user to enter the value ofn
. - We use the
scanf
function to read the value ofn
entered by the user and store it in then
variable. - We declare a for loop that runs from 0 to infinity. Inside the loop, we call the
isArmstrong
function to check whether the current number is an Armstrong number or not. If it is an Armstrong number, we increment thecount
variable. The loop stops whencount
becomes equal ton
. - We use the
printf
function to display the Nth Armstrong number on the screen. - We declare the
isArmstrong
function that takes an integernum
as input and returns an integer as output. Inside the function, we declare four integer variablesoriginalNum
,remainder
,n
, andresult
, and initialize theresult
variable to 0. - We store the value of
num
inoriginalNum
variable for future reference. - We use a while loop to calculate the number of digits in the number
num
and store it in then
variable. - We use another while loop to calculate the sum of nth power of each digit of the number
num
and store it in theresult
variable. - We check whether the number
num
is an Armstrong number or - not by comparing the value of
result
with the value ofnum
. If they are equal, then the function returns 1, which indicates that the number is an Armstrong number. Otherwise, the function returns 0, indicating that the number is not an Armstrong number. - Conclusion:
- In this program, we have successfully written a C program to find the Nth Armstrong number. We have used a function to check whether a number is Armstrong or not. This program can be useful in various applications where we need to find the Nth Armstrong number.