This C program calculates the area of a trapezium based on the user’s input of the lengths of its two bases and the height.
Problem statement
Write a C program that prompts the user to enter the lengths of the bases and the height of a trapezium. The program should then calculate and display the area of the trapezium.
C Program to Find Area of Trapezium
#include <stdio.h> int main() { float base1, base2, height, area; printf("Program to calculate the area of a trapezium\n"); printf("Enter the length of base 1: "); scanf("%f", &base1); printf("Enter the length of base 2: "); scanf("%f", &base2); printf("Enter the height: "); scanf("%f", &height); area = (base1 + base2) * height / 2; printf("The area of the trapezium is: %.2f\n", area); return 0; }
How it works
- The program prompts the user to enter the lengths of base 1, base 2, and the height of the trapezium.
- The values are stored in the variables
base1
,base2
, andheight
respectively. - The program calculates the area using the formula
(base1 + base2) * height / 2
and stores it in the variablearea
. - Finally, the program displays the calculated area using
printf
with the format specifier%.2f
to display the result with two decimal places.
Input / output
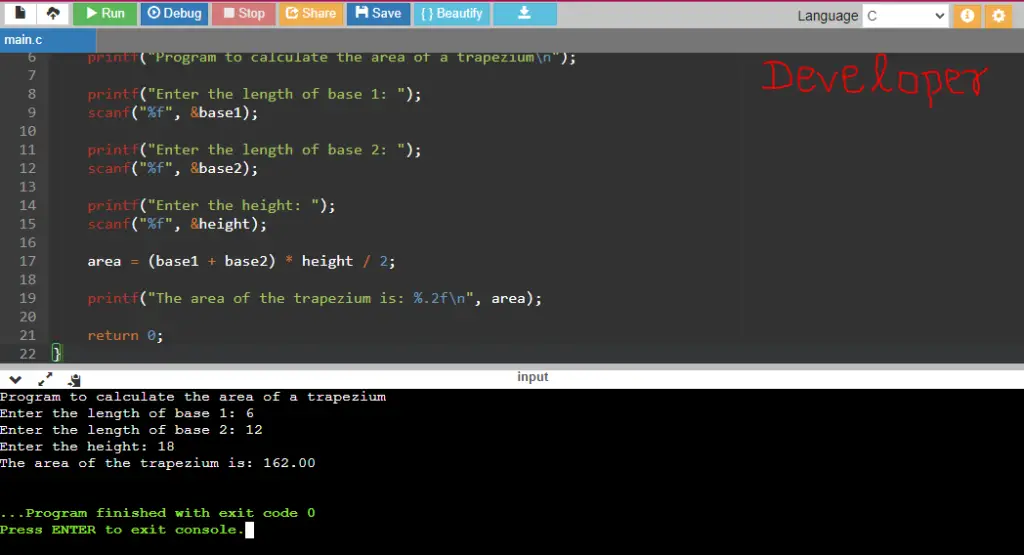