In this post, you will learn about the C program that takes two strings as input and determines whether they are anagrams or not. An anagram is a word or phrase formed by rearranging the letters of another word or phrase. In other words, if two strings contain the same characters in the same quantities, but in a different order, they are considered anagrams.
Problem Statement
Your task is to write a C program that takes two strings as input and determines whether they are anagrams or not.
Your program should have the following specifications:
- Prompt the user to enter the first string.
- Prompt the user to enter the second string.
- Compare the characters and their frequencies in the two strings to determine if they are anagrams.
- Output an appropriate message indicating whether the strings are anagrams or not.
Ensure that your program handles strings of different lengths and is case-sensitive, meaning that uppercase and lowercase letters are considered distinct.
C Program to Check whether two Strings are Anagrams
#include <stdio.h> #include <string.h> #define MAX_SIZE 100 int checkAnagram(char str1[], char str2[]); int main() { char str1[MAX_SIZE], str2[MAX_SIZE]; printf("Enter the first string: "); gets(str1); printf("Enter the second string: "); gets(str2); if (checkAnagram(str1, str2)) printf("The strings are anagrams.\n"); else printf("The strings are not anagrams.\n"); return 0; } int checkAnagram(char str1[], char str2[]) { int len1 = strlen(str1); int len2 = strlen(str2); // Anagrams must have the same length if (len1 != len2) return 0; // Count the occurrence of each character in str1 int count[256] = {0}; for (int i = 0; i < len1; i++) count[(int)str1[i]]++; // Decrement the count for each character in str2 // If a character count goes below 0, the strings are not anagrams for (int i = 0; i < len2; i++) { count[(int)str2[i]]--; if (count[(int)str2[i]] < 0) return 0; } return 1; }
How it Works?
The C program to check whether two strings are anagrams works based on the following steps:
- The program prompts the user to enter the first string and reads it from the standard input.
- Similarly, the program prompts the user to enter the second string and reads it from the standard input.
- The program calls the
checkAnagram
function, passing the two input strings as arguments. - The
checkAnagram
function first compares the lengths of the two strings. If they are different, it immediately returns 0, indicating that the strings are not anagrams. - Next, the function creates an integer array
count
of size 256 and initializes all its elements to 0. This array is used to count the occurrence of each character in the first string. - The function then iterates over each character of the first string and increments the count for that character in the
count
array. - After counting the characters in the first string, the function iterates over each character of the second string. For each character, it decrements the count for that character in the
count
array. - If the count for any character in the
count
array becomes negative during the iteration of the second string or if a character is encountered in the second string that is not present in the first string, the function immediately returns 0, indicating that the strings are not anagrams. - If the function completes the iteration of the second string without encountering any issues, it returns 1, indicating that the strings are anagrams.
- Back in the
main
function, the program checks the return value of thecheckAnagram
function. If it is 1, the program prints the message “The strings are anagrams.” Otherwise, it prints the message “The strings are not anagrams.”
That’s how the program works to determine whether two strings are anagrams or not. By comparing the characters and their frequencies, it accurately identifies whether the strings can be rearranged to form the same word or phrase.
Input / Output
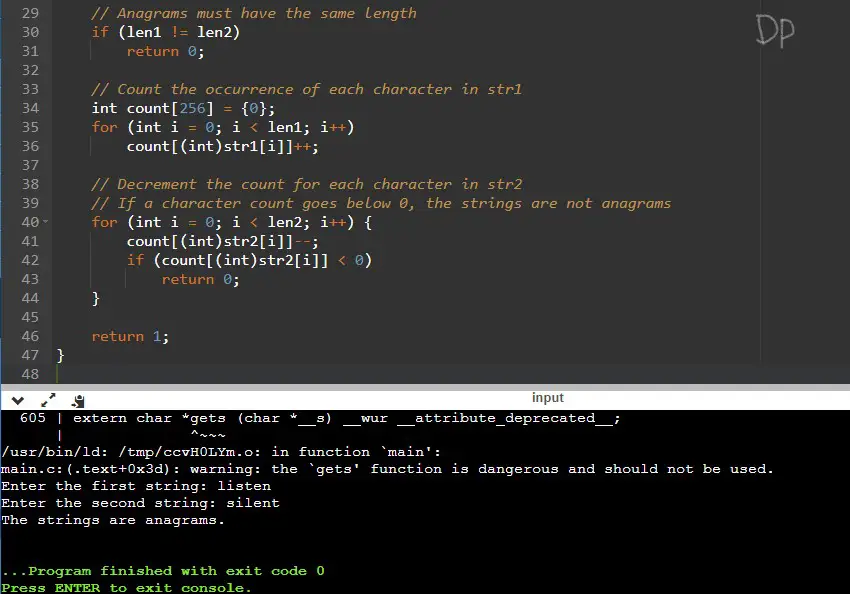