In programming, it is often necessary to convert characters from lowercase to uppercase and vice-versa. In this program, we will write a C program that takes a string as input and converts all the lowercase characters to uppercase and vice-versa.
Problem Statement
The problem statement is to write a C program that takes a string as input and converts all the lowercase characters to uppercase and vice-versa.
Solution
Here is the C program to convert lowercase characters to uppercase and vice-versa:
#include <stdio.h> #include <ctype.h> int main() { char str[100]; int i; printf("Enter a string: "); gets(str); i = 0; while (str[i]) { if (islower(str[i])) { putchar(toupper(str[i])); } else if (isupper(str[i])) { putchar(tolower(str[i])); } else { putchar(str[i]); } i++; } return 0; }
Output
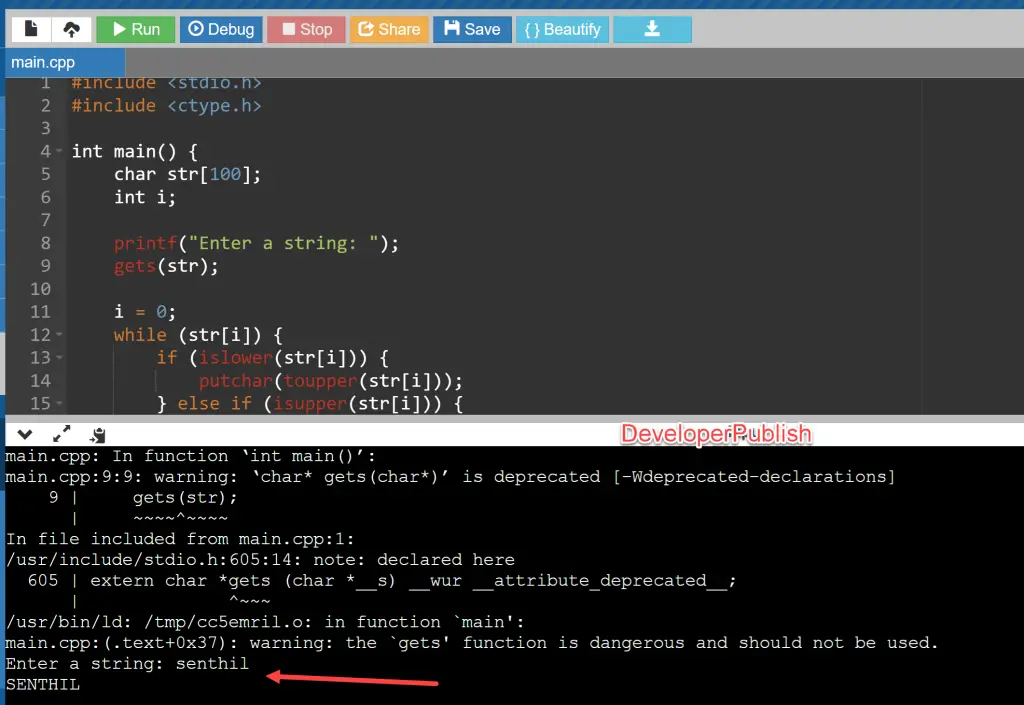
Explanation
First, we include the stdio.h
header file which is used for input/output functions, and the ctype.h
header file which is used for character handling functions. Then, we define the main()
function as the starting point of our program.
We declare a character array str
which will be used to store the string entered by the user, and an integer variable i
which will be used to iterate through the string.
Next, we prompt the user to enter a string using the printf()
function. The gets()
function is used to read in the string entered by the user.
We use a while
loop to iterate through the string. Within the loop, we check whether the current character is a lowercase or uppercase character using the islower()
and isupper()
functions respectively. If it is a lowercase character, we convert it to uppercase using the toupper()
function and print it using the putchar()
function. If it is an uppercase character, we convert it to lowercase using the tolower()
function and print it using the putchar()
function. If it is neither a lowercase nor an uppercase character, we simply print it using the putchar()
function.
Finally, we return 0 to indicate successful program execution.
Conclusion
In this tutorial, we learned how to write a C program to convert lowercase characters to uppercase and vice-versa. We used the islower()
, isupper()
, toupper()
, tolower()
, and putchar()
functions to achieve this.