This Python program validates a given date input by the user. It checks whether the entered date is a valid date according to the Gregorian calendar.
Problem statement
You are required to write a program that verifies the validity of a date entered by the user. The program should ensure that the date is within the acceptable range and adheres to the correct format.
Python Program to Validate a Date
def is_valid_date(year, month, day): # Check if the year is within a valid range if year < 1 or year > 9999: return False # Check if the month is within a valid range if month < 1 or month > 12: return False # Check if the day is within a valid range if day < 1 or day > 31: return False # Check for months with less than 31 days if (month == 4 or month == 6 or month == 9 or month == 11) and (day > 30): return False # Check for February and leap years if month == 2: if day > 29: return False if day == 29 and (year % 4 != 0 or (year % 100 == 0 and year % 400 != 0)): return False # Date is valid return True # Get user input date_str = input("Enter a date (YYYY-MM-DD): ") # Extract year, month, and day from the input string year, month, day = map(int, date_str.split('-')) # Validate the date if is_valid_date(year, month, day): print("The entered date is valid.") else: print("The entered date is not valid.")
How it works
- The program defines a function
is_valid_date
that takes three arguments:year
,month
, andday
. This function checks various conditions to determine if the date is valid or not. - The function first checks if the year is within the range of 1 to 9999. If not, it returns
False
. - Next, it checks if the month is within the range of 1 to 12. If not, it returns
False
. - Then, it checks if the day is within the range of 1 to 31. If not, it returns
False
. - If the month is April (4), June (6), September (9), or November (11), the function checks if the day is greater than 30. If so, it returns
False
since these months have a maximum of 30 days. - For February (2), the function checks if the day is greater than 29. If so, it returns
False
. Additionally, if the day is exactly 29, it checks if the year is not a leap year, returningFalse
in such cases. - If none of the above conditions are met, the function returns
True
, indicating that the date is valid. - The program prompts the user to enter a date in the format “YYYY-MM-DD” and stores it in the
date_str
variable. - The program uses the
split
method to extract the year, month, and day from the input string and converts them to integers. - Finally, the program calls the
is_valid_date
function with the extracted year, month, and day as arguments. If the function returnsTrue
, it prints “The entered date is valid.” Otherwise, it prints “The entered date is not valid.”
Input / Output
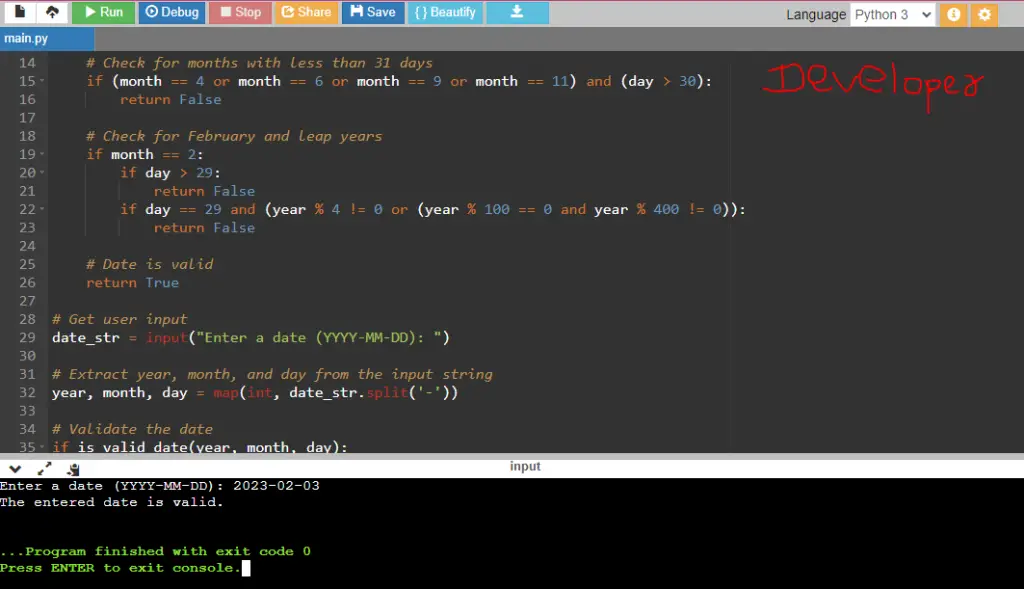