This Python program swaps adjacent nodes in a circular linked list. A circular linked list is a linked list where the last node points back to the first node. Swapping adjacent nodes means exchanging the positions of two consecutive nodes in the list.
Swapping adjacent nodes in a circular linked list involves exchanging the positions of nodes that are next to each other within the list. A circular linked list is a data structure in which each node points to the next node, and the last node connects back to the first node, forming a closed loop. Swapping adjacent nodes can be a useful operation when reordering elements or modifying the arrangement of data within the circular linked list.
Problem Statement
You are given a circular linked list, where each node contains an integer value. Implement a function that swaps the data of adjacent nodes throughout the circular linked list.
Python Program to Swap Adjacent Nodes in a Circular Linked List
class Node: def __init__(self, data): self.data = data self.next = None class CircularLinkedList: def __init__(self): self.head = None def append(self, data): new_node = Node(data) if not self.head: self.head = new_node new_node.next = self.head else: temp = self.head while temp.next != self.head: temp = temp.next temp.next = new_node new_node.next = self.head def display(self): if not self.head: return temp = self.head while True: print(temp.data, end=" ") temp = temp.next if temp == self.head: break print() def swap_adjacent_nodes(self): if not self.head or not self.head.next: return current = self.head while current.next != self.head: current.data, current.next.data = current.next.data, current.data current = current.next.next # Example usage cll = CircularLinkedList() cll.append(1) cll.append(2) cll.append(3) cll.append(4) print("Original Circular Linked List:") cll.display() cll.swap_adjacent_nodes() print("\nCircular Linked List after swapping adjacent nodes:") cll.display()
How it Works
- Initial Circular Linked List:markdownCopy code
1 -> 2 -> 3 -> 4 ^ | |_________________|
- Identify and Swap Adjacent Nodes:
- Start at the first node (1).
- Swap the data of the current node (1) with the data of the next node (2).
- Move to the next node (2).
- Swap the data of the current node (2) with the data of the next node (3).
- Move to the next node (3).
- Swap the data of the current node (3) with the data of the next node (4).
- Move to the last node (4).
- Swap the data of the current node (4) with the data of the next node (1).
- The entire circular linked list has been traversed, and adjacent nodes have been swapped.
- Updated Circular Linked List:
As a result of swapping adjacent nodes, the circular linked list changes its arrangement. The values 1 and 2 were swapped, as well as the values 3 and 4. The circular structure of the linked list is maintained, and the data within the nodes is modified.
It’s important to note that this swapping process focuses on the data contained within the nodes. The structure of the linked list itself—how the nodes are connected through pointers or references—is not altered. This is why it’s called “swapping adjacent nodes” rather than “swapping adjacent pointers.”
Input/ Output
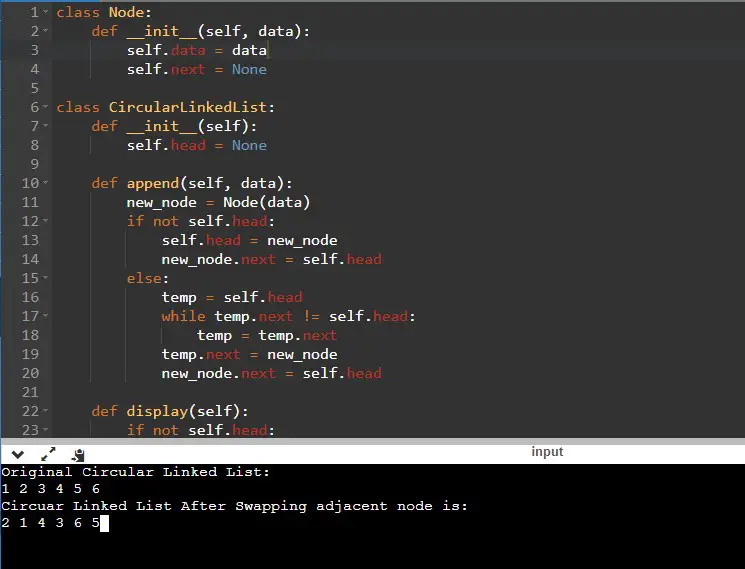